Images are a crucial part of web material in the modern world. They aid in telling stories, evoking feelings, and capturing readers’ interest. You might need to download several photographs online if you’re a developer, marketer, or content creator or anybody who has a constant need for visual data.
Thankfully, Python comes with strong modules that make this task simple. We’ll look at the finest Python methods for image downloading using Python in this blog post. With Python being over 30 years old, it has achieved 22% yearly growth.
We’ll review the fundamentals of downloading photos from websites to your computer and even more complex strategies like multi-threading and resizing. After going through this article, you’ll thoroughly understand how to use Python to automate image-downloading chores.
Setting Up Your Environment to Download Images Using Python
To download images of web pages from the internet, you need to be setting up your environment. Following are some general guidelines:
- Download Compatible Python Version
Python’s latest can be downloaded and installed from the official website. Select the version that is compatible with your operating system.
- Install the Required Software
Several libraries for obtaining images are available in Python. Requests, BeautifulSoup, urllib, and urllib2 are frequently used packages. Pip is a Python package manager that may be used to install these items. To install the packages, open the command prompt and execute pip install.
- Virtualize Your Environment
Virtualizing your environment is a great way to keep the dependencies for your project and the system apart. Open the command prompt and execute python -m venv env-name> to establish a virtual environment. Download the virtual environment library.
pip install venv
Now, you need to create a new environment in your working directory’s path by using this command:
python -m venv /path/to/new/virtual/environment_name
Now that you have created the environment, you need to be in it by simply activating it.
If you’re using Ubuntu or Mac, try this:
source <venv_name>/bin/activate
If you’re using Windows, try this:
C:\> <venv_name>\Scripts\activate
Since the virtual environment is now setup, you just need to download the libraries needed to get started. Install the libraries as needed and run this command to save all those libraries in a file so you don’t have to manually install them the next time:
pip freeze > requirements.txt
The purpose of your environment is to make things safe and sound from external interference of libraries that are not needed to run the project. Python images downloading process can be started after your environment has been configured.
The next time when you run the
pip install -r requirements.txt
Download Images Using Python
To retrieve images from a website using Python. Here’s an example
Code:
1 | from urllib2 import urlopen |
This is what the code outputs look like.
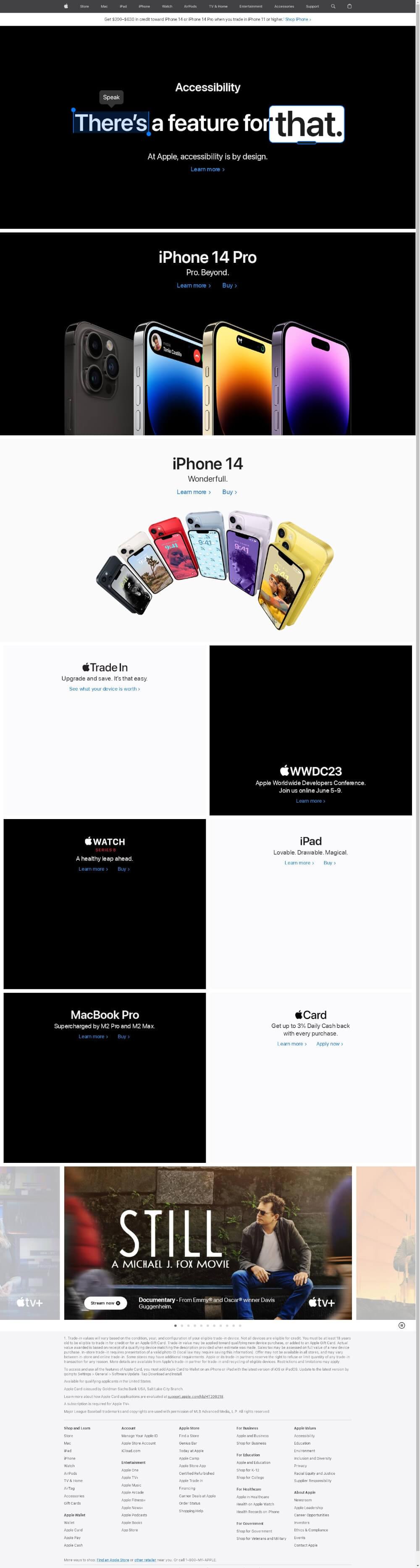
You may quickly manage and organize the downloaded photographs on your computer by setting up directories and file paths.
Advanced Image-Downloading Tips & Techniques
Here are a few advanced techniques about how you can download images :
- Multi-threading: You can utilize multi-threading to speed up the process of downloading many images. You can download numerous images at once using multi-threading.
- Resizing photos: Resize images you download to a specified size before using them. To resize photos, you can use Python tools like Pillow or OpenCV.
- Image filtering: If you’re pulling images from more than one source, you can sort them according to the file type, resolution, or color. Use computer vision libraries like OpenCV or scikit-image to filter images based on their content and Python libraries like imghdr or Pillow to filter photos based on their file format.
- Error handling: When downloading numerous photos, handling problems reasonably is necessary. You can utilize Python’s try-except blocks to handle exceptions like HTTP problems, file I/O issues, and network errors. The logging library can record information for debugging and recording mistakes.
- Using a web crawler: You can use a web crawler for Python, like Scrapy or Beautiful Soup, to have images from numerous online pages but that requires a lot of error handling and building a vast flow of processes as well as managing the infrastructure to be able to do all that. Using a web crawler, you may automate browsing across numerous pages and obtaining pictures with just URLs. A web crawler can also filter photos based on particular standards and download them concurrently utilizing multi-threading.
- Verify for duplications: Look for duplicates to prevent wasting storage space and bandwidth. Python tools like hashlib or imghdr can search for duplicate files based on file type or content.
Best Practices for Python Image Downloading
It’s crucial to adhere to best practices while downloading images using Python or a web crawler to ensure the procedure is quick, dependable, and moral. The following are some recommendations to remember:
- Respect website policies: Read the terms of service and copyright guidelines before downloading any photos. While some websites may expressly forbid the automated downloading or scraping of their content, others could demand credit or permission before being used commercially.
- Use caching and rate limitation techniques: Limit the number of requests per second and avoid repeatedly requesting the same content to avoid overloading a website’s server and getting blacklisted. To implement caching and rate limitation, you can utilize Python libraries like requests-cache and rate-limit.
- Handle exceptions and errors: When downloading photos, be ready to handle exceptions and errors, including HTTP, connection timeouts, and file I/O problems. To handle exceptions, utilize try-except blocks, log errors, and debug information.
- Optimise image quality and size: To reduce file size and speed up page loads, consider optimizing the image quality and size while downloading photos. Python libraries like Pillow or OpenCV can resize and compress photos.
- Attribute and cite sources: Attribute and cite the sources appropriately when using images downloaded from other websites. You can use metadata or watermarks to add attribution information to the images.
What Libraries Can I Use To Download Images In Python?
Python has built-in libraries like urllib and urllib2 and third-party libraries like requests, BeautifulSoup, and Scrapy. This can be long, hectic process. Simply, use Screenshots API and take screenshots of any web page you like in a matter of seconds and get them in an organized structure, ready to be stored as needed.
How Do I Handle Exceptions When Downloading Images?
Use try-except blocks to handle exceptions like HTTP, connection timeouts, and file I/O errors. Screenshots API lets you pass all those headaches onto the developers working behind the infrastructure so you just have to worry about what you need and not how you need it.
Can I Download Multiple Images Simultaneously In Python?
Asynchronous programming or multi-threading can be used to download several photos at once. You can make about 20 requests per second to take full page, high-resolution screenshots at a time.
How Can I Check For Duplicates When Downloading Images?
You can use Python libraries like hashlib or imghdr to check for duplicates based on file content or file type.
Can I Download Images From A Password-protected Website Using Python?
You can use Python’s built-in authentication mechanisms or third-party libraries like requests_ntlm or requests_oauthlib to authenticate and download images. Alternatively, you can pass in parameters with the Screenshots API which would do the job just right for you.
How Can I Download Images From An API Using Python?
You can use Python’s requests library to make API requests and download images from the response. You can also use the API along with Screenshots API which would anonymously get the visual data for you.
Is It Legal To Download Images From The Web Using Python?
It depends on the website’s terms of service and copyright policies. Always read the website’s policies and follow ethical and legal guidelines when taking screenshots or downloading images.
Conclusion
Crawlbase’s Screenshots API can be used as a practical tool to automate the gathering and analysis of enormous amounts of visual data. Whether you’re building a machine learning model, analyzing social media trends, or collecting photos for a personal project, Screenshots API offers a flexible and efficient environment for collecting images. Python can be a very functional language for this purpose.
We covered the fundamentals of downloading images with Python in this blog post, along with some tips and best practices to help you speed up the process. You can quickly and confidently download photos by preparing your environment, identifying image sources, resolving exceptions and mistakes, and utilizing sophisticated techniques like multi-threading and image optimization.
As always, it’s essential to respect website policies, attribute and cite sources, and follow ethical and legal guidelines when downloading images from the web. By following these principles effectively, you can unlock the power of Python image download and take your projects to the next level.