Apple App Store is a digital marketplace where users can discover, download, and install applications for Apple devices such as iPhones and iPads. It contains a diverse range of apps, including games, productivity tools, and entertainment apps. Currently hosting millions of apps, the App Store serves as a vast repository of software created by developers worldwide. Data within the App Store includes app names, descriptions, user reviews, ratings, and download statistics.
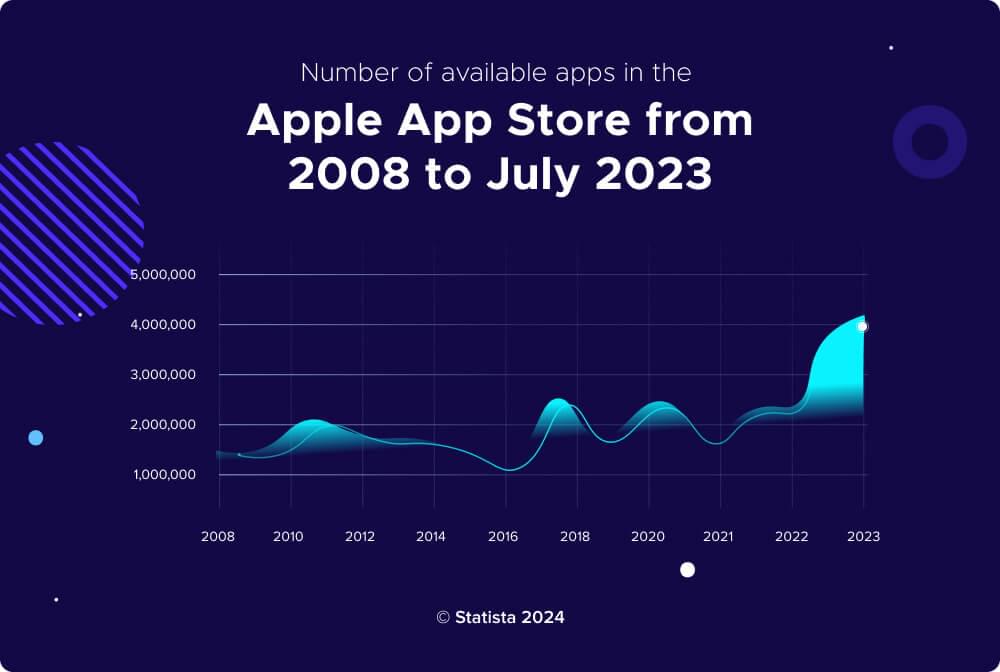
In this tutorial, we’ll explore how to scrape data from the Apple App Store using the Crawlbase Crawling API and JavaScript. These tools are essential for gathering various types of data, such as app rankings, reviews, and descriptions, from the store’s pages. The Apple App Store has a lot of useful information for developers, marketers, and researchers looking to analyze trends, track app performance, and understand user preferences. With the right apple app store scraper and techniques, anyone interested in app analytics or market research can use the Apple App Store’s data to make smart decisions and find valuable insights.
If you want to get right into scraping apple app store data, click here.
Table of Contents
- Apple App Store Insights
- Why Scrape Apple App Store
- Prerequisites
- Scrape Apple App Store Data
- Fetching the HTML
- How to Scrape App Data
- Final Thoughts
- Frequently Asked Questions
Apple App Store Insights: Rankings, Reviews, Descriptions
When it comes to scrape data from the Apple App Store, it’s super important for developers, marketers, and researchers. This part of the guide talks about three main kinds of data you can scrape from the App Store: App Rankings, Reviews and Ratings, and App Descriptions.
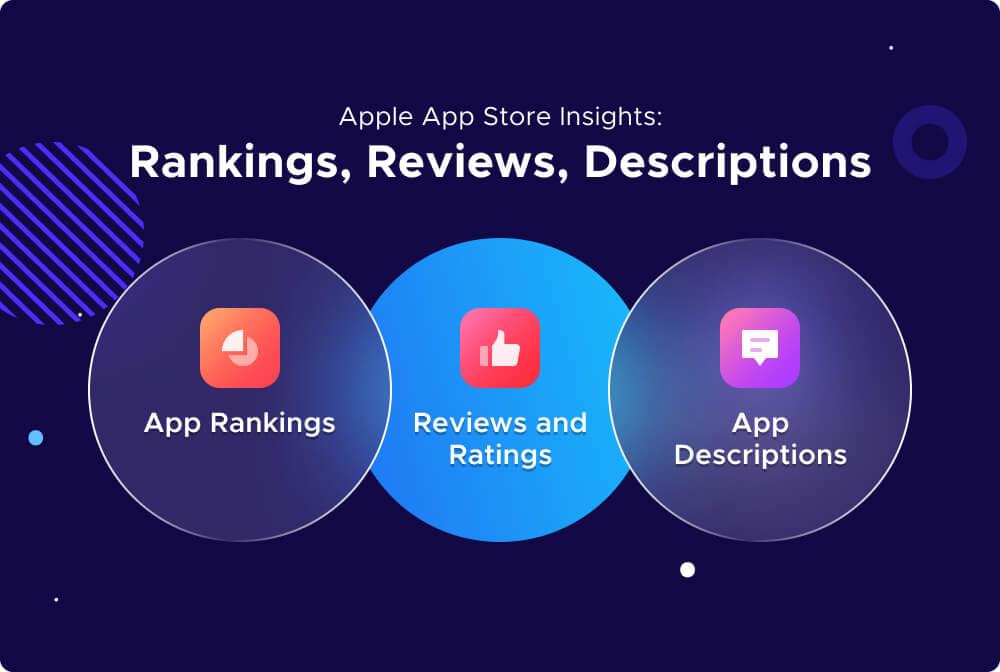
App Rankings
App rankings show how popular and well an app is doing in the App Store. When you scrape this data, you can see which apps are getting more attention or becoming less popular. It helps them make smart decisions about how to make their apps better and keep up with what users want. By understanding these trends, developers can improve their apps and stay competitive in the busy world of app stores.
Reviews and Ratings
Reviews and ratings play a pivotal role in users’ decision-making processes. Scraping this data allows developers and marketers to gauge user satisfaction, identify areas for improvement, and respond to user feedback. By analyzing sentiments expressed in reviews and correlating them with ratings, stakeholders can gain a comprehensive understanding of an app’s strengths and weaknesses. This information is instrumental in refining marketing strategies, addressing user concerns, and ultimately fostering a positive user experience.
App Descriptions
Scraping app descriptions provides insights into how developers position their apps in the marketplace. Understanding how competitors articulate their app’s features and benefits can inform developers’ own marketing strategies. Additionally, app descriptions often include keywords that contribute to an app’s discoverability within the App Store search algorithm. By analyzing these descriptions, marketers can optimize their app’s metadata to enhance visibility and attract a broader audience.
Why Scrape Apple App Store
Scraping data from the Apple App Store holds significant value for developers, marketers, and researchers alike. Each group benefits uniquely from the wealth of information that can be extracted from this digital marketplace.
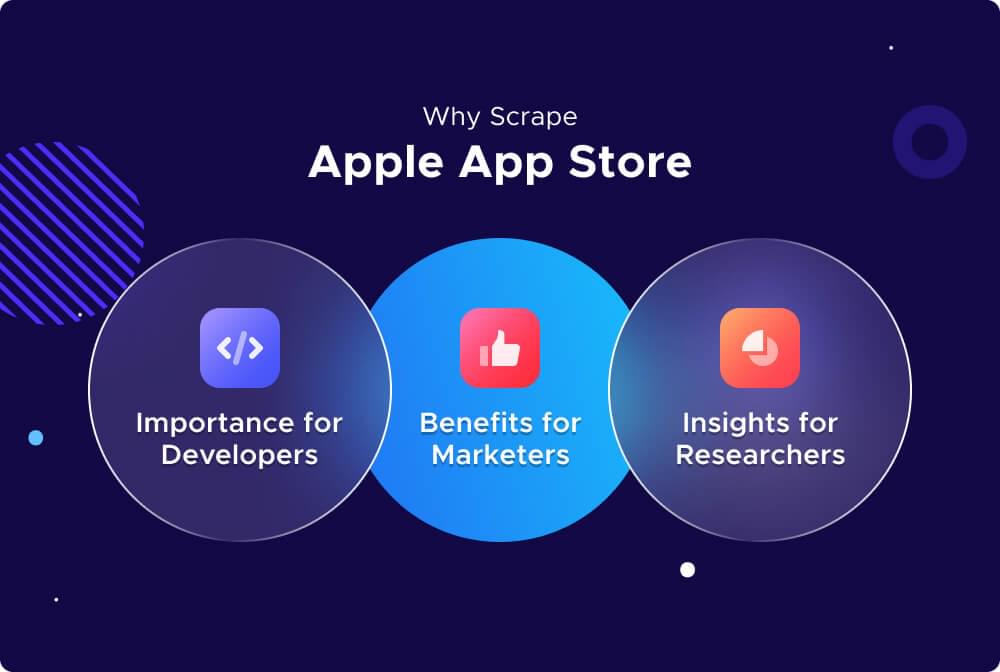
- Importance for Developers
For developers, scraping the Apple App Store is like looking at the heart of the app world. If they understand how well an app is ranked, it tells them how much people like it compared to other apps. This information is super important for developers who want to make their apps better. By knowing what users like, developers can make changes, fix problems, and keep their apps interesting in a changing market. The data they get from scraping is like a guide, helping developers figure out how to make their apps better and keep them successful.
- Benefits for Marketers
Marketers gain a lot by scraping the Apple App Store because it shows how competitors present their apps. When they look at app descriptions, marketers get a guide on how to create interesting stories for their own apps. By knowing what language and features users like, marketers can make their messages more attractive. Also, by using the keywords from app descriptions, marketers can make sure their app shows up more in searches, reaching more people and getting more downloads. Scraping the Apple App Store becomes a powerful tool for marketers, helping them talk to users effectively and get more people interested in their apps.
- Insights for Researchers
Apple App Store scraping is a big help for researchers. It gives them a lot of data to study trends and how users behave. By putting together reviews, ratings, and app rankings, researchers can see patterns and what people like. This info is super useful for understanding how mobile apps are changing, what users feel, and the new trends in the market. Researchers can find connections, pick out unusual things, and create detailed insights. These insights can be used in academic studies, market reports, and industry analysis. The data they get from scraping is like a goldmine for researchers studying the mobile app world.
Prerequisites
Before you start writing code, make sure you have a few things ready:
- Node.js on your computer:
Node.js helps you run JavaScript on your computer, and it’s important for our web scraping script. Get Node.js by downloading and installing it from the official Node.js website.
- Basic understanding of JavaScript:
Since we’re using JavaScript for web scraping, it’s good to know some basics. This includes things like understanding variables, functions, loops, and basic DOM manipulation. If you’re new to JavaScript, check out beginner tutorials or read the guides on websites like Mozilla Developer Network (MDN) or W3Schools.
- Crawlbase API Token:
We’ll use the Crawlbase Crawling API for efficient web scraping. To make it work, you need an API token. Create an account on the Crawlbase website, and in your account settings, find your API tokens. These tokens act like keys to unlock the features of the Crawling API.
Scrape Apple App Store Data
Let’s prepare your tools for the JavaScript code. Follow these steps:
Create Project Folder:
Open your terminal and enter mkdir app_store_scraper
to make a new project folder.
mkdir app_store_scraper
Navigate to Project Folder:
Type cd app_store_scraper
to enter the new folder and make it easier to handle your project files.
cd app_store_scraper
Create JavaScript File:
Enter touch scraper.js
to create a new file named scraper.js
(you can pick a different name if you prefer).
touch scraper.js
Install Crawlbase Package:
Type npm install crawlbase
to add the Crawlbase tool to your project. This tool is crucial as it helps you connect with the Crawlbase Crawling API, simplifying the process of gathering information from the Apple App Store’s website.
npm install crawlbase
By following these steps, you’re setting up everything you need for your Apple App Store scraping project. You’ll have a specific folder, a JavaScript file for your code, and the necessary Crawlbase tool for an organized and efficient scraping process.
Fetching the HTML
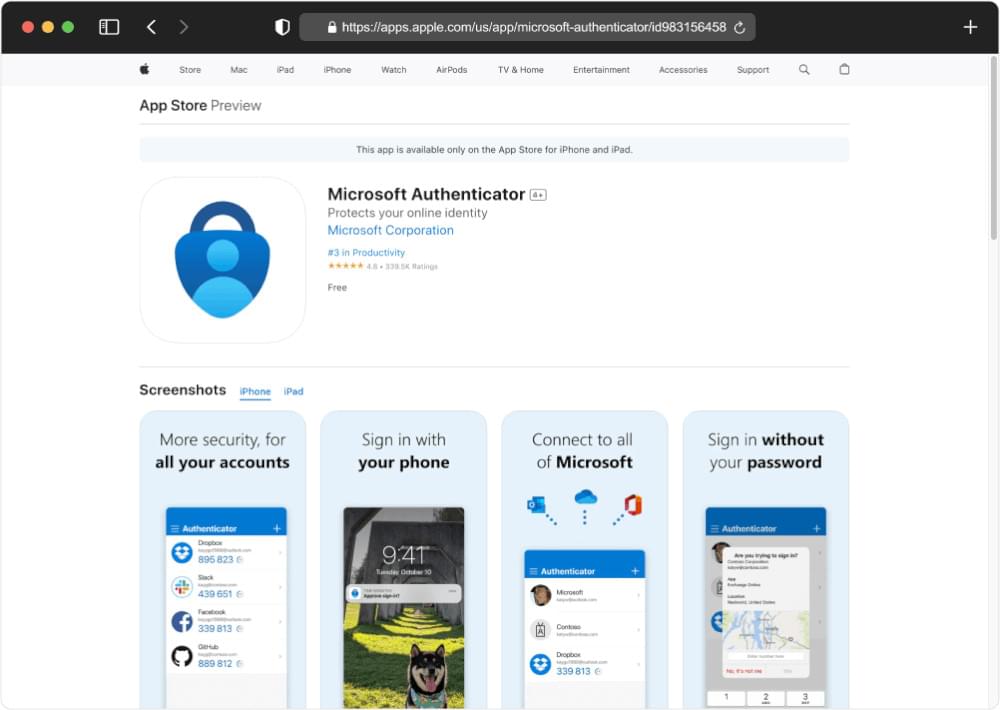
Once you have your API credentials and the Node.js library for web scraping installed, we can begin working on the “scraper.js” file. Pick the Apple App Store app you want to scrape data from – for example, let’s focus on the Microsoft Authenticator App. In the “scraper.js” file, use Node.js, Crawlbase Crawling API and the fs library to scrape data from the chosen Apple App Store page. Make sure to change the placeholder URL in the code with the actual URL of the page you want to scrape.
1 | const { CrawlingAPI } = require('crawlbase'), |
The above code snippet uses the Crawlbase library to crawl HTML content from the Apple App Store page of the application. The script starts by setting up a Crawling API instance with a token, then it sends a GET request to the Apple App Store page. If the response is successful with a status code of 200, it saves the HTML content into a file named “response.html”. If there are any errors during the crawling, it prints the error message to the console.
HTML Response:
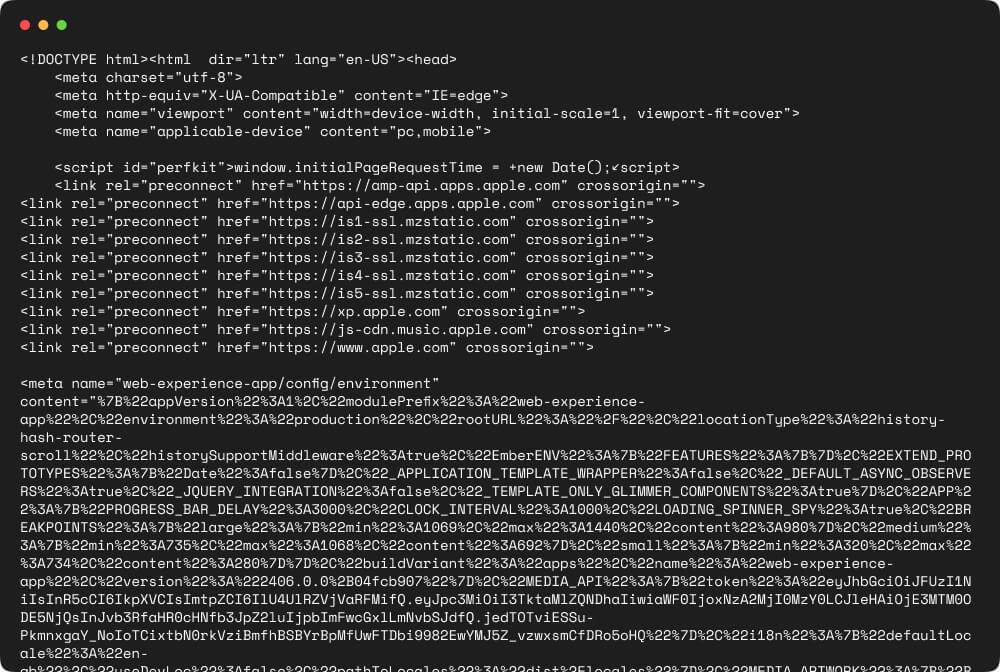
How to Scrape App Data
In this part, we’re going to find out how to scrape important and useful data from an Apple App Store application. The details we aim to scrape include things like the app title, subtitle, seller, image, stars, rating, price, description, reviews, size, category and more. To achieve this, we’ll create a special JavaScript scraper using two libraries: cheerio, commonly used for web scraping, and fs, which helps with file operations. The provided script will analyze the HTML code of the Apple App Store page (which we got in the previous example), pick out the required information, and store it in a JSON array.
1 | const fs = require('fs'), |
JSON Response:
1 | { |
Final Thoughts
This detailed guide provides you with everything you need to scrape data from the Apple App Store using JavaScript and the Crawlbase Crawling API. It helps you scrape various details from the Apple App Store, such as app logos, titles, subtitles, seller info, prices, star ratings, user reviews, and descriptions. Whether you’re new to web scraping or already have some experience, these tips and tricks are here to make your journey easier and help you succeed in extracting data effectively.
Explore additional guides that might interest you:
How to Scrape Yellow Pages Data
How to Scrape Alibaba Search Results Data
How to Scrape Movie Data from IMDb
How to Scrape News Articles from Bloomberg
If you need help or face any challenges during your scraping journey, the Crawlbase support team is available to assist you. Your success in web scraping is important to us, and we’re here to support you every step of the way.
Frequently Asked Questions
Which app store scraper is best?
The Crawlbase Crawling API is a great choice as apple app store scraper. It’s easy to use and has clear instructions. JavaScript, a flexible programming language, is often used to get dynamic content from the App Store. Because it can handle modern web applications well, thanks to its asynchronous features and compatibility with browsers. By using Crawlbase and JavaScript together, developers can easily scrape important data from the Apple App Store for analysis and understanding.
Does Apple have an API?
Yes, there is an API available for accessing Apple App Store data. One prominent API is the iTunes Search API provided by Apple. This API allows developers to search for content within the App Store, including apps, movies, music, and more. With the iTunes Search API, developers can retrieve information such as app details, user reviews, ratings, and pricing. By integrating this API into their applications or scripts, developers can access and utilize Apple App Store data in a structured and efficient manner for various purposes.
Can I scrape Apple App Store data without getting blocked?
To scrape Apple App Store data without getting blocked, you need to act like a human and avoid being detected. The Crawlbase Crawling API helps with this by rotating IPs, changing user-agent strings, solving CAPTCHAs, avoiding traps, acting like a human, saving cookies, and hiding signs of automation. These features reduce the chances of being blocked, making sure your data extraction runs smoothly. Crawlbase Crawling API is designed to improve the effectiveness of web scraping projects while keeping things ethical and legal, making it less likely for your activities to be detected and blocked.
Can web scraping monitor App Store ranking changes?
Yes, web scraping can be employed to monitor changes in App Store rankings effectively. By extracting and analyzing data regularly, developers can track the performance and positions of apps over time. This information helps businesses and app developers stay informed about market trends, competitors, and the effectiveness of marketing strategies. Implementing a scraping solution allows for timely adjustments to improve app visibility and rankings in response to dynamic market conditions.