IMDb, or Internet Movie Database, is a popular online hub filled with details about movies, TV shows, and more. With over 17.86 million movie titles, 13.14 million human records, and 83 million registered users, it’s a vast database. Think of it as a massive library where you can explore films, actors, directors, and trivia. Whether you’re a movie buff or a researcher, IMDb is the go-to source for analyzing digital content. It’s the perfect place for effortless data scraping, offering valuable insights to anyone interested in entertainment.
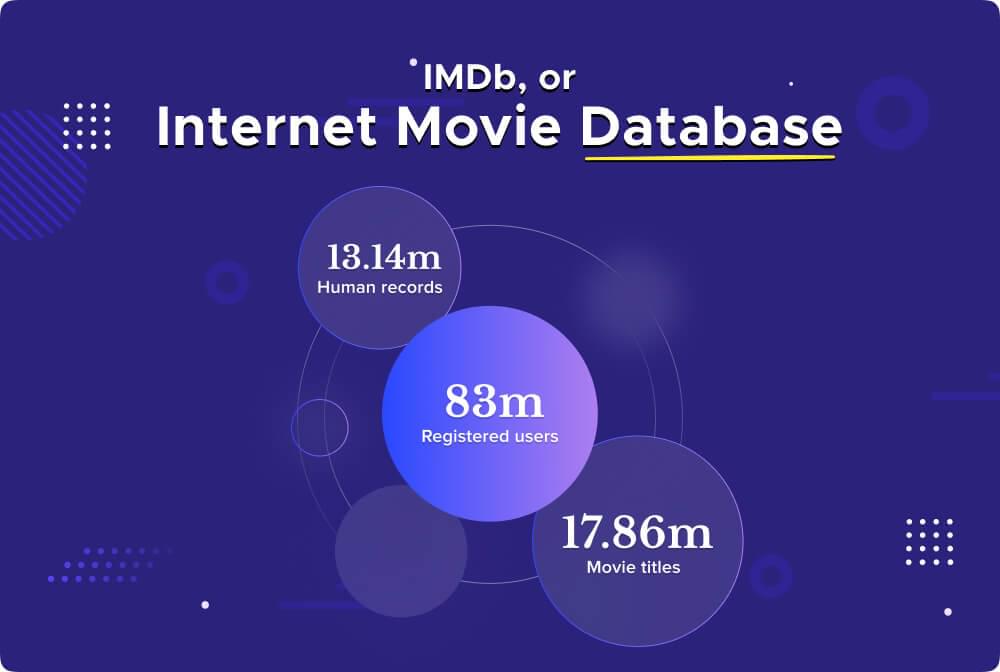
In this tutorial, we’ll explore how to extract IMDb movies data using the Crawlbase Crawling API and JavaScript. Using these tools, we’ll easily scrape movie data like movie title, rating, release date, duration, cast, crew, storyline, genre, and more. Unlocking IMDb’s secrets becomes simple, letting you gather comprehensive information for your cinematic journey. Join us in navigating the digital landscape, using Crawlbase Crawling API and JavaScript to scrape and reveal the rich details within IMDb’s vast database.
Table of Contents
- Why Scrape IMDb Movie Data
- IMDb Data Structure
- Prerequisites
- How to Build an IMDb Scraper
- Fetch the HTML Content
- Scrape IMDb Movie Data
- Extract IMDb Movie Insights with Crawlbase
- Frequently Asked Questions
Why Scrape IMDb Movie Data
In the digital era, scraping IMDb movie data brings numerous benefits, unlocking insights and possibilities for movie lovers, researchers, and content creators. This process provides valuable information efficiently, empowering users to explore the cinematic world with ease and discover trends, preferences, and new opportunities in the realm of movies.
- Automation for Efficient Data Retrieval
Scraping IMDb data programmatically offers a key advantage – automation. This means you can automatically fetch movie details without the hassle of manual data collection. It’s like having a tireless assistant that tirelessly gathers information, saving you valuable time and effort.
- Real-Time Updates for Latest Information
One significant perk of programmatic access to IMDb is the ability to receive real-time updates. Whether it’s about newly released movies or the latest ratings, scraping allows you to stay on the pulse of the ever-evolving movie landscape. Keep your data fresh and up-to-date without any manual intervention.
- Customization Tailored to Your Needs
Programmatic access provides the flexibility to customize your data retrieval process. Want information on specific genres, release years, or other criteria? With scraping, you can tailor the process to your preferences, creating a personalized dataset that aligns perfectly with your interests or research goals.
- Content Aggregation for Comprehensive Databases
Scraped IMDb data finds practical applications in content aggregation. By building a comprehensive database of movie details, you can contribute to the creation of services that offer users an easy solution for all their movie-related queries. It’s about bringing together a wealth of information into a cohesive and accessible resource.
- Insights and Analytics for Informed Decision-Making
Analyzing IMDb data opens doors to valuable insights. Identify trends in popular genres, understand the influence of actors and directors on ratings and box office performance, and uncover patterns that contribute to a film’s success or failure. These insights empower filmmakers, content creators, and researchers to make informed decisions in the dynamic world of movies.
IMDb Data Structure
IMDb’s comprehensive data structure acts as the backbone for movie enthusiasts, researchers, and content creators seeking detailed insights into the world of films.
Movie Title and Basics:
IMDb encapsulates fundamental details, starting with movie titles, release dates, and durations. This foundational information provides a quick overview for users navigating the vast cinematic landscape.
Ratings and Audience Feedback:
One of IMDb’s prominent features is its rating system. Users can explore audience ratings, providing an immediate gauge of a movie’s popularity and reception.
Cast and Crew Lists:
Delving deeper, IMDb meticulously categorizes the individuals contributing to a film’s creation. Cast lists highlight actors’ roles, while crew details encompass directors, writers, producers, and more, providing a comprehensive understanding of the talent behind the scenes.
Storyline and Synopsis:
For those seeking a glimpse into a movie’s narrative, IMDb offers concise storylines and synopses. This feature serves as a valuable resource for users interested in the plot without revealing too much.
Genre Classification:
Genres play a pivotal role in categorizing movies. IMDb’s data structure ensures accurate genre classification, aiding users in discovering films aligned with their preferences.
Additional Details and Trivia:
IMDb goes beyond the basics, offering trivia, goofs, and additional details that enrich the user experience. These tidbits provide interesting insights into the filmmaking process and enhance overall engagement.
Awards and Recognitions:
For a comprehensive view of a movie’s acclaim, IMDb includes information on awards won or nominations received. This section acknowledges the industry recognition garnered by a film and its contributors.
Prerequisites
Before you start coding, make sure you have the following things ready:
Node.js on your computer:
Node.js is a tool that lets you run JavaScript on your computer. It’s important for running the web scraping script we’re going to create. Download and install Node.js from the official website Node.js.Basic understanding of JavaScript:
Since we’re using JavaScript for web scraping, it’s important to know the basics of the language. This includes understanding variables, functions, loops, and basic DOM manipulation. If you’re new to JavaScript, check out introductory tutorials or documentation on websites like Mozilla Developer Network (MDN) or W3Schools.Crawlbase API Token:
We’ll be using Crawlbase Crawling API for efficient web scraping. The API token is needed to verify your requests. Go to the Crawlbase website, create an account, and find your API tokens in your account settings. These tokens act as keys to unlock the features of the Crawling API.
How to Build an IMDb Scraper
Let’s get your tools ready for the JavaScript code. Here’s what you need to do:
- Create Project Folder:
Open your terminal and typemkdir imdb_scraper
to create a new project folder.
mkdir imdb_scraper
- Navigate to Project Folder:
Typecd imdb_scraper
to go into the new folder and make it easier to manage your project files.
cd imdb_scraper
- Create JavaScript File:
Typetouch scraper.js
to make a new file named scraper.js (you can choose a different name if you want).
touch scraper.js
- Install Crawlbase Package:
Typenpm install crawlbase
to add the Crawlbase tool to your project. This tool is important because it helps you communicate with the Crawlbase Crawling API, making it easier to gather information from IMDb’s website.
npm install crawlbase
By following these steps, you’re getting everything ready for your IMDb scraping project. You’ll have a dedicated folder, a JavaScript file for your code, and the necessary Crawlbase tool for organized and efficient scraping.
Fetch the HTML Content
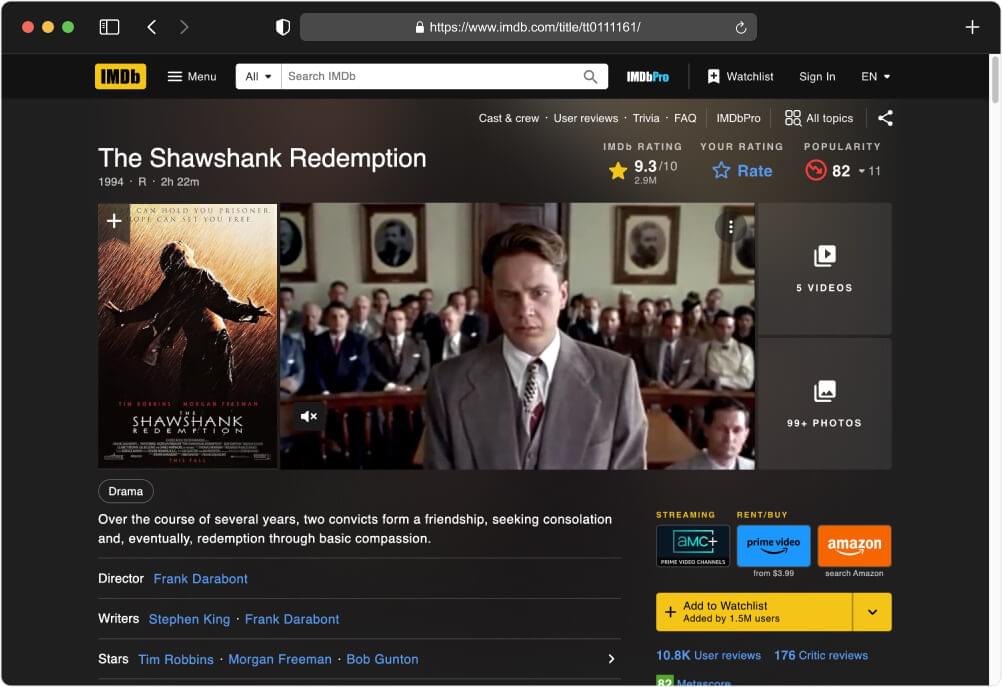
Now that you have your API credentials and the Node.js library for web scraping installed, let’s start working on the “scraper.js” file. Pick the IMDb movie you want to scrape data from – for this example, let’s focus on The Shawshank Redemption (1994). In the “scraper.js” file, use Node.js and the fs library to extract data from the chosen IMDb movie page. Remember to replace the placeholder URL in the code with the actual URL of the page you want to scrape.
1 | const { CrawlingAPI } = require('crawlbase'), |
The above code snippet utilizes the Crawlbase library to scrape HTML content from the IMDb page of the movie. The script initializes a CrawlingAPI
instance with a token, makes a GET request to the IMDb page, and upon a successful response with a status code of 200, it writes the HTML content to a file named “response.html”. In case of any errors during the crawling process, it logs the error to the console.
HTML Response:
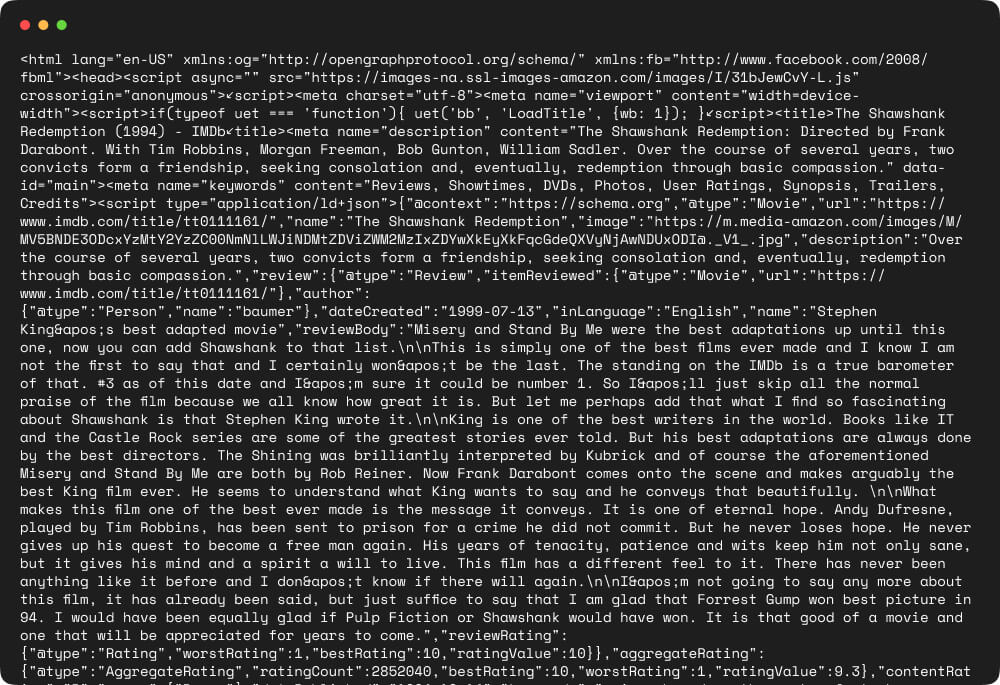
Scrape IMDb Movie Data
In this section, we’ll learn how to scrape important meaningful data from an IMDb movie page. The data we want to scrape includes details like the movie title, rating, release date, duration, cast, crew, story line, genre, and more. To do this, we’ll build a special JavaScript scraper using two libraries: cheerio, often used for web scraping, and fs, which helps with file operations. The script we provide will analyze the HTML code of the IMDb page (which we fetched in the previous example), extract the needed information, and save it in a JSON array.
1 | const fs = require('fs'), |
The provided JavaScript code uses the cheerio
library to parse and extract information from an HTML file of IMDb page. The script reads the HTML content from the “response.html” file, loads it into a Cheerio instance, and then employs various selectors and extraction functions to gather data.
The extracted movie data includes the title, IMDb rating, genre, plot outline, director, writers, stars, user reviews, critic reviews, metascore, release date, country of origin, language, and production company. The information is organized into a movieData
object and printed as a formatted JSON string
JSON Response:
1 | { |
Extract IMDb Movie Insights with Crawlbase
This guide provides you with information and tools to assist in scraping data from IMDb using JavaScript and the Crawlbase Crawling API. You can collect diverse sets of data, including movie titles, ratings, release dates, duration, cast, crew, storyline, genre, and more. Whether you’re a beginner in web scraping or have some experience, these tips will help you begin. If you’re keen on trying scraping on other websites like Bloomberg, Product Hunt, or Expedia, we have additional guides for you to explore.
Related Guides:
Frequently Asked Questions
Can I scrape movie data from IMDb?
Web scraping is generally considered legal, but specific platforms may have rules you must follow. IMDb permits the use of its content for non-personal purposes, but you should review IMDb’s Conditions of Use for detailed regulations. It’s crucial to be mindful of your data usage and comply with your country’s laws. While limited scraping for personal, non-commercial use may be tolerated. Extensive or commercial-scale scraping of IMDb data is forbidden without explicit permission. Additionally, some movies/TV shows may have copyright restrictions that prohibit scraping.
Are there frequency limitations for IMDb scraping?
IMDb does not officially disclose specific frequency limitations for scraping its site. However, it is advisable to follow ethical scraping practices, avoid overloading their servers, and consider their terms of service. To simplify this process, consider using the Crawlbase Crawling API, which provides a structured and managed approach to web scraping. This API allows users to fetch data at controlled intervals, ensuring compliance with website policies and preventing excessive requests that could lead to IP bans.
How to handle dynamic content when scraping IMDb?
When scraping dynamic content on IMDb, the Crawlbase Crawling API is a valuable tool. It efficiently handles JavaScript-generated pages, including those built with React, Angular, Vue, Ember, Meteor, etc. The API ensures accurate data extraction by crawling and providing the full HTML content even if it relies heavily on dynamic scripting. This feature allows users to successfully scrape IMDb’s dynamic content, capturing comprehensive information while benefiting from the simplicity and effectiveness of the Crawlbase Crawling API.
Is IMDb API Free?
IMDb does not provide an official public API for accessing its data. However, there are unofficial APIs and third-party services that offer access to IMDb’s data in various formats, such as JSON or XML. These unofficial APIs may have limitations and may not be endorsed by IMDb. It’s important to review their terms of service and usage policies before integrating them into your projects for accessing IMDb data. As an additional solution, consider the Crawlbase Crawling API, a structured web scraping tool, ensuring a compliant and efficient approach to accessing IMDb data.