TikTok scraping is becoming the utmost demand of businesses in 2024 because TikTok has quickly become a big deal in social media, grabbing people’s attention with its fun short and interactive videos. With over 1 billion monthly active users globally and millions of videos uploaded daily, TikTok has become a warehouse of valuable data ripe for exploration and analysis. Lots of people love using it, which makes it a great place for advertisers, scientists, and software creators who want to learn about what’s popular, how people act, and what trends are taking off.
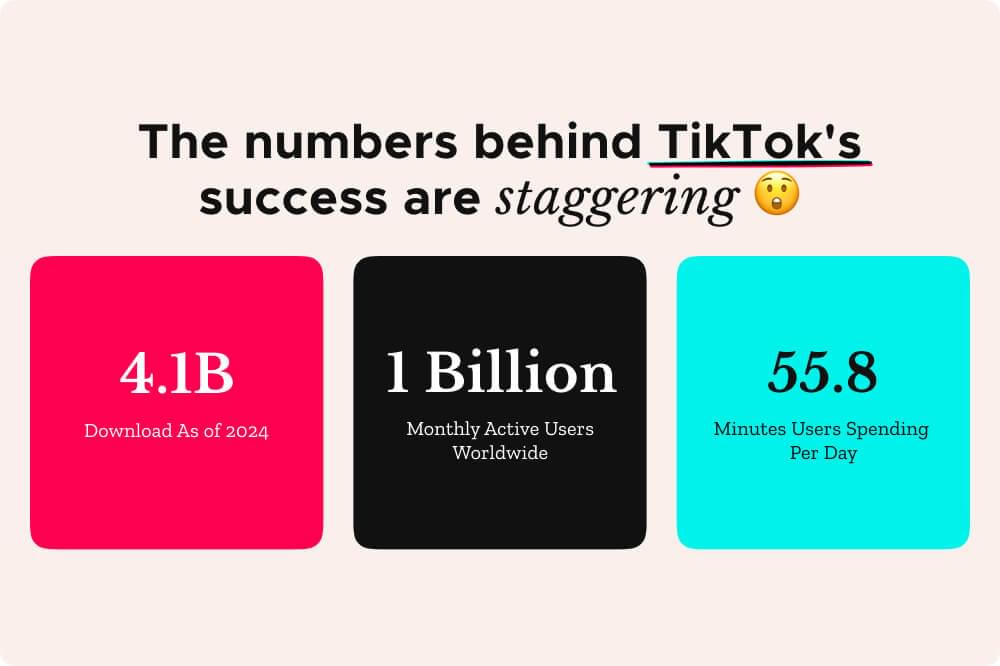
The numbers behind TikTok’s success are staggering. TikTok has been downloaded more than 4.1 billion times. As of 2024, TikTok boasts a user base of over 1 billion monthly active users worldwide, surpassing other leading social media platforms in terms of engagement and content consumption. The platform garners billions of video views daily, with users spending an average of 55.8 minutes per day browsing through their personalized feeds. Moreover, TikTok’s search volume has skyrocketed, with millions of users actively seeking out content across a wide range of topics, from entertainment and lifestyle to education and DIY tutorials.
In this comprehensive guide, we’ll be scraping TikTok using Python and the Crawlbase Crawling API. You will learn how to scrape tiktok followers, videos and more. We’ll walk you through the process of extracting HTML content, scraping search results, handling pagination, and saving data for further analysis.
Table Of Contents
- Project Scope
- Prerequisites
- Project Setup
- Extracting TikTok Page HTML
- Scraping TikTok Search Listing
- Scraping TikTok Video Details
- Scraping TikTok Video Author Details
- Scraping TikTok Video Hashtags
- Complete Code - TikTok Scraper
- Handling Pagination in TikTok Scraper
- Saving Scraped TikTok Data into a CSV File
- Final Thoughts
- Frequently Asked Questions (FAQs)
- What is TikTok Scraping?
- Why Scrape TikTok?
- Is It Legal to Scrape TikTok?
- What Can You Scrape from TikTok?
- What are the Best Ways to Scrape TikTok?
1. Project Scope
In this guide, our objective is to provide a user-friendly tutorial on scraping TikTok using Python and the Crawlbase Crawling API. Our project focuses on first getting the HTML content using usual methods. Then, we’ll see the problems with these methods. After that, we’ll use the Crawlbase Crawling API to solve these issues. Alongside, we’ll use Python’s BeautifulSoup library to effectively understand and collect data from TikTok.
We’ll primarily focus on scraping various elements from TikTok, including video details, author information, hashtags from search results. Our aim is to present a step-by-step approach that caters to users with diverse technical backgrounds.
Key Components of the Project:
- HTML Crawling: We’ll leverage Python alongside the Crawlbase Crawling API to fetch the complete HTML content of TikTok pages. This approach ensures thorough data extraction while adhering to TikTok’s usage policies. We’ll target TikTok SERP.
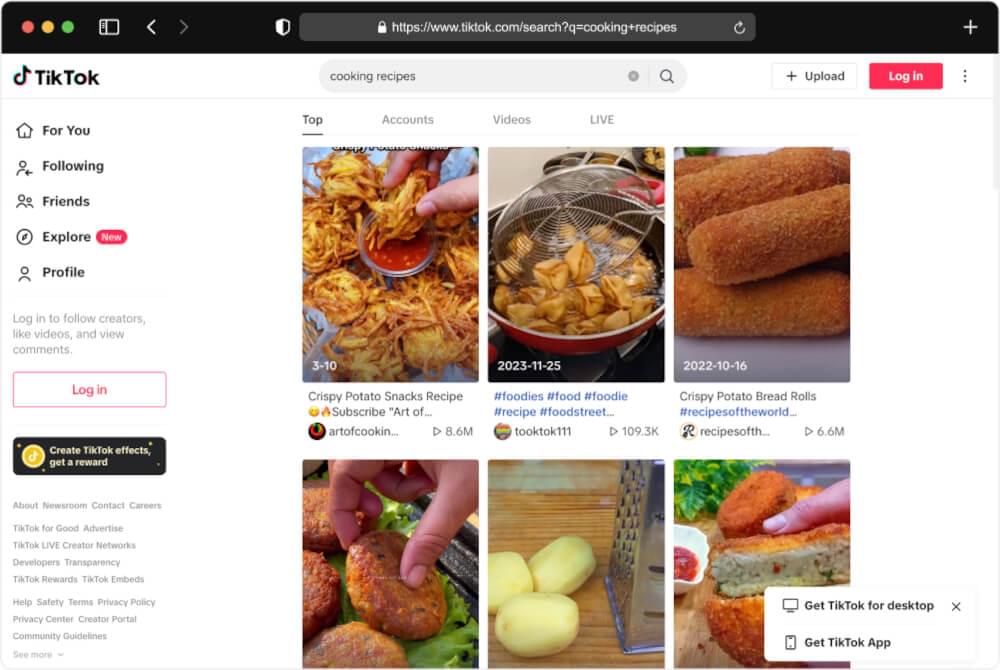
- Data Extraction from TikTok: Our primary focus will be on using BeautifulSoup in Python to extract specific data elements from TikTok pages. This includes scraping video details, author information, and hashtags for all search results.
- Handling Pagination: To navigate through multiple pages of TikTok results, we’ll discuss the pagination mechanisms employed by TikTok. This ensures that all relevant data is captured during the scraping process.
- Saving Data: We’ll explore methods to store or save the scraped data, offering options such as saving to a CSV file for further analysis.
By outlining the project scope, our aim is to guide you through a comprehensive TikTok scraping tutorial, making the process accessible and achievable. Let’s now proceed to the prerequisites of the project.
2. Prerequisites
Before delving into the realm of web scraping TikTok with Python, it’s essential to ensure you have the necessary prerequisites in place:
- Basic Python Knowledge: Familiarize yourself with the Python programming language, as it will be used to write scripts for scraping TikTok data. Understanding concepts like variables, loops, and functions will be beneficial.
- Create Crawlbase Account: Sign up for a Crawlbase account and obtain your API tokens. One of these tokens are required to get authenticated with Crawling API. You can get your tokens here after signing up. First 1,000 requests are free of cost. No Credit Card required!
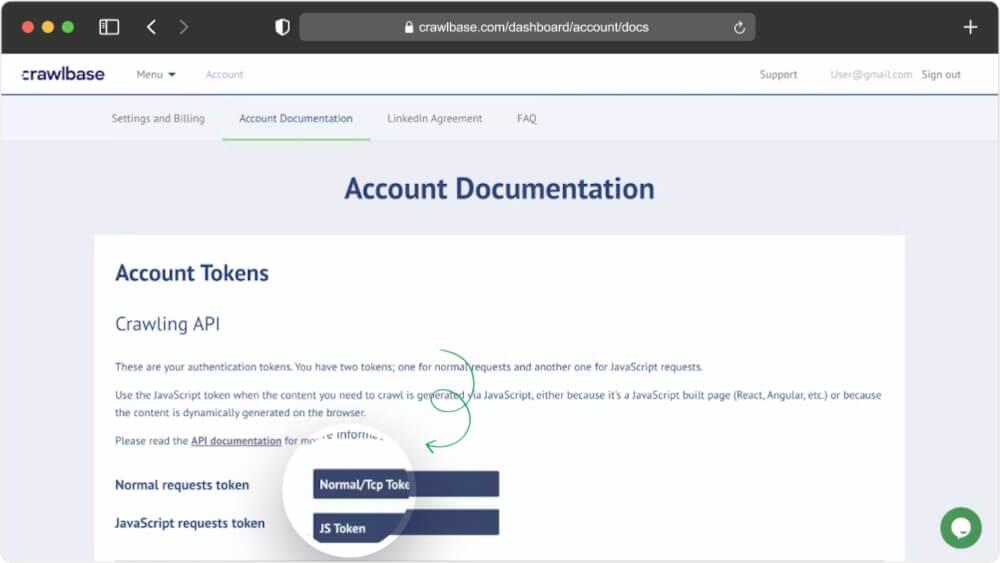
- Choosing a Token: Crawlbase provides two types of tokens – Normal Token tailored for static websites and JS Token designed for dynamic or JavaScript-driven websites. TikTok relies heavily on JavaScript rendering, so we will use JS Token.
- Python Installation: You can download Python from the official Python website based on your operating system. Additionally, confirm the presence of pip (Python package manager), which usually comes bundled with Python installations.
1 | # Use this command to verify python installation |
By fulfilling these prerequisites, you’ll be ready to embark on your TikTok scraping journey with confidence and efficiency.
3. Project Setup
To kickstart your TikTok scraping project, follow these steps to set up your development environment.
Create a New Python Environment
Start by creating a new Python environment for your project. You can use virtual environments to keep your project dependencies separate from other Python projects. Use the following command to create a new virtual environment named “tiktok-env”:
1 | python -m venv tiktok-env |
Activate the Virtual Environment
Once the virtual environment is created, activate it using the appropriate command for your operating system:
For Windows:
1
tiktok-env\Scripts\activate
For macOS and Linux:
1
source tiktok-env/bin/activate
Install Required Libraries
With the virtual environment activated, install the necessary Python libraries for web scraping. Use pip to install the following libraries:
1 | pip install requests beautifulsoup4 pandas crawlbase |
- Requests: For sending HTTP requests to TikTok’s servers.
- BeautifulSoup4: For parsing HTML content retrieved from TikTok pages.
- Pandas: For data manipulation and analysis.
- Crawlbase: For accessing TikTok pages efficiently using the Crawling API.
Set Up Crawlbase API Credentials
Ensure you have obtained your Crawlbase API credentials, including your access token. You’ll need these credentials to authenticate and access TikTok pages via the Crawlbase Crawling API.
Initialize Your Python Script
Create a new Python script file tiktok_scraper.py
for your TikTok scraping project. You can use any text editor or integrated development environment (IDE) of your choice to write your Python code.
By following these setup steps, you’ll have a fully configured development environment ready to begin scraping data from TikTok. This setup ensures a smooth and efficient workflow as you proceed with your scraping project.
4. Extracting TikTok Page HTML
When scraping TikTok, the first step is to retrieve the HTML content of the page you are targeting. There are different approaches to accomplish this task, each with its own set of challenges and considerations.
Extracting HTML Using Common Approach
The common approach to extracting HTML from TikTok involves sending HTTP requests directly to TikTok’s servers. This can be achieved using Python libraries like Requests
for sending requests.
1 | import requests |
Copy above code into your tiktok_scraper.py file and run the following command in the directory where file is present.
1 | python tiktok_scraper.py |
You will see that the HTML of the page get presented on the terminal.
But why the is no useful information in the HTML? It’s because TikTok relies on JavaScript rendering to load essential data dynamically. Unfortunately, with conventional scraping methods, accessing this data can be challenging. TikTok’s anti-scraping measures further complicate the process. As a result, scraping TikTok using traditional approaches may not yield satisfactory results.
Challenges While Scraping TikTok Using Common Approach
Scraping TikTok using the common approach presents several challenges, including:
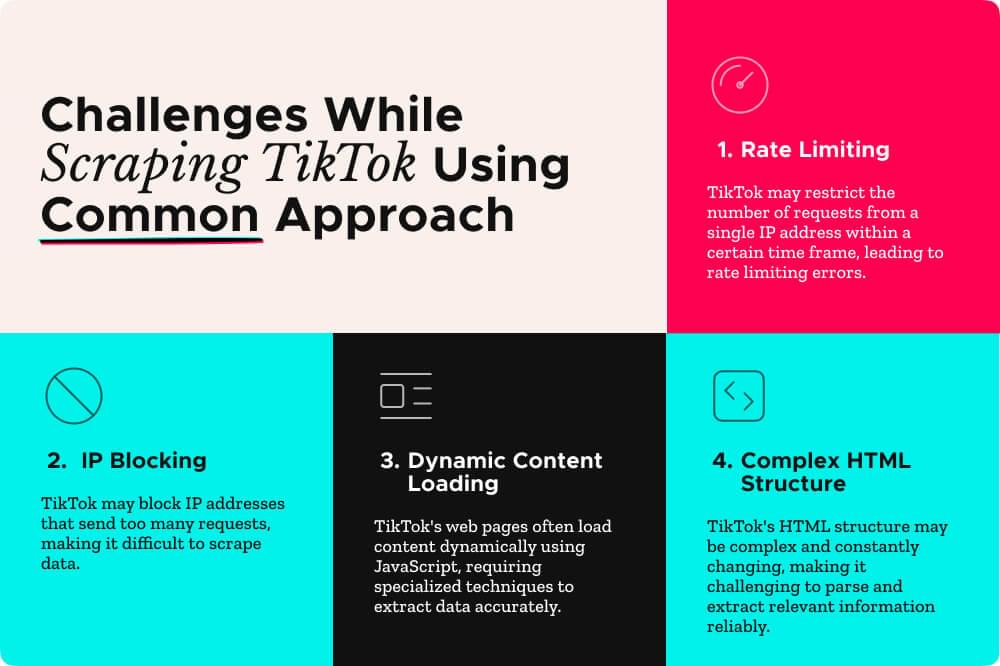
- Dynamic Content Loading: TikTok’s web pages often load content dynamically using JavaScript, requiring specialized techniques to extract data accurately.
- Rate Limiting: TikTok may restrict the number of requests from a single IP address within a certain time frame, leading to rate limiting errors.
- IP Blocking: TikTok may block IP addresses that send too many requests, making it difficult to scrape data. While there are ways to scrape websites without getting blocked, the best one is to use a TikTok scraper.
- Complex HTML Structure: TikTok’s HTML structure may be complex and constantly changing, making it challenging to parse and extract relevant information reliably.
To overcome these obstacles, we’ll use a smarter method with the help of the advanced features provided by the Crawlbase Crawling API.
Extracting HTML Using Crawlbase Crawling API
An alternative approach to extract HTML from TikTok is to leverage the Crawlbase Crawling API. Crawlbase provides a reliable and efficient way to access TikTok pages programmatically while overcoming common scraping challenges. Its parameters allow you to handle any kind of scraping problem with ease.
To overcome the JS rendering issue, we can use ajax_wait and page_wait parameters provided by Crawling API. Below is an example which uses Crawlbase library to access Crawling API and send a request to fetch tiktok page HTML along with required parameters.
1 | from crawlbase import CrawlingAPI |
Example Output:
Using the Crawlbase Crawling API simplifies the scraping process and allows you to focus on extracting valuable data from TikTok with ease.
5. Scraping TikTok Search Listing
Once we have extracted the HTML content of the TikTok search results page, the next step is to scrape specific data elements from the search results
We’ll begin by extracting the search listing, which includes all the search results displayed on the TikTok search page.
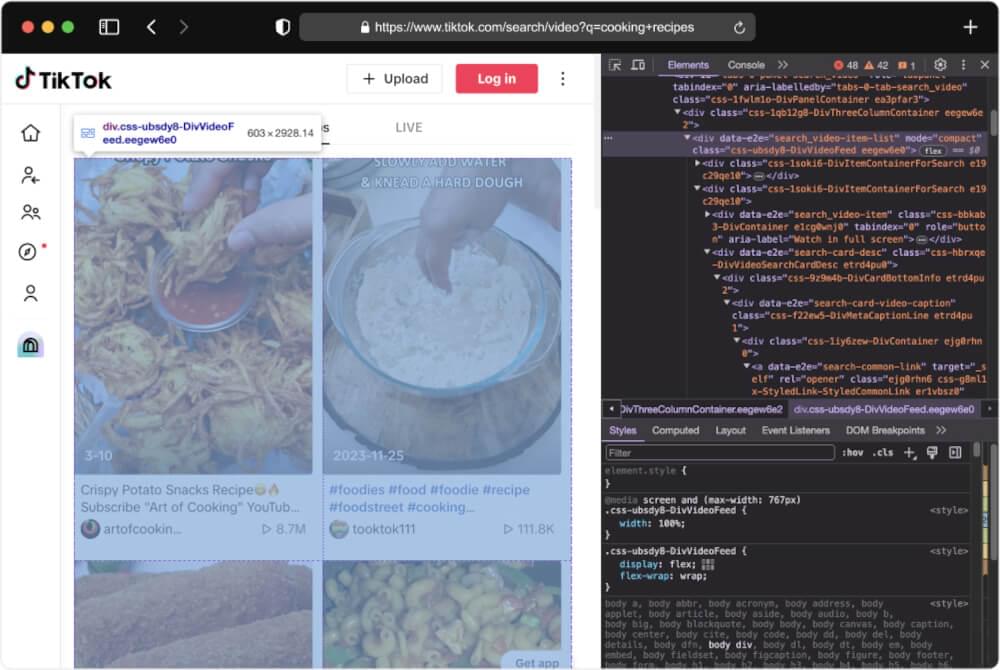
1 | from crawlbase import CrawlingAPI |
6. Scraping TikTok Video Details
To scrape TikTok video details such as video caption, video url, thumbnail url, upload date, and views count, we’ll need to locate the HTML elements containing this information.
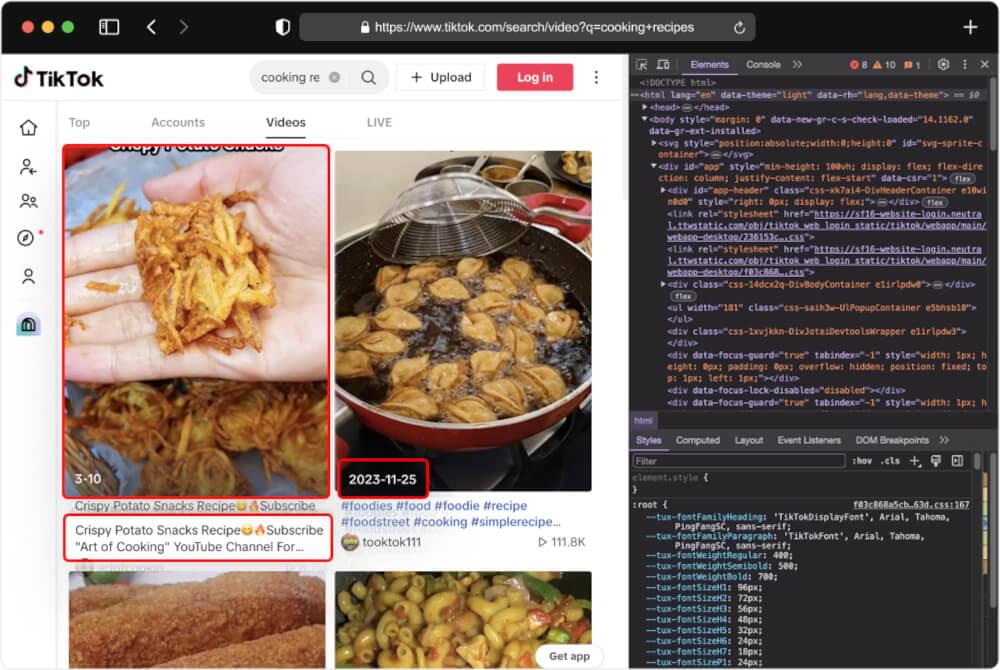
1 | # Function to scrape video details |
7. Scraping TikTok Video Author Details
We can extract author information such as user name, profile url, and image url from each video card.
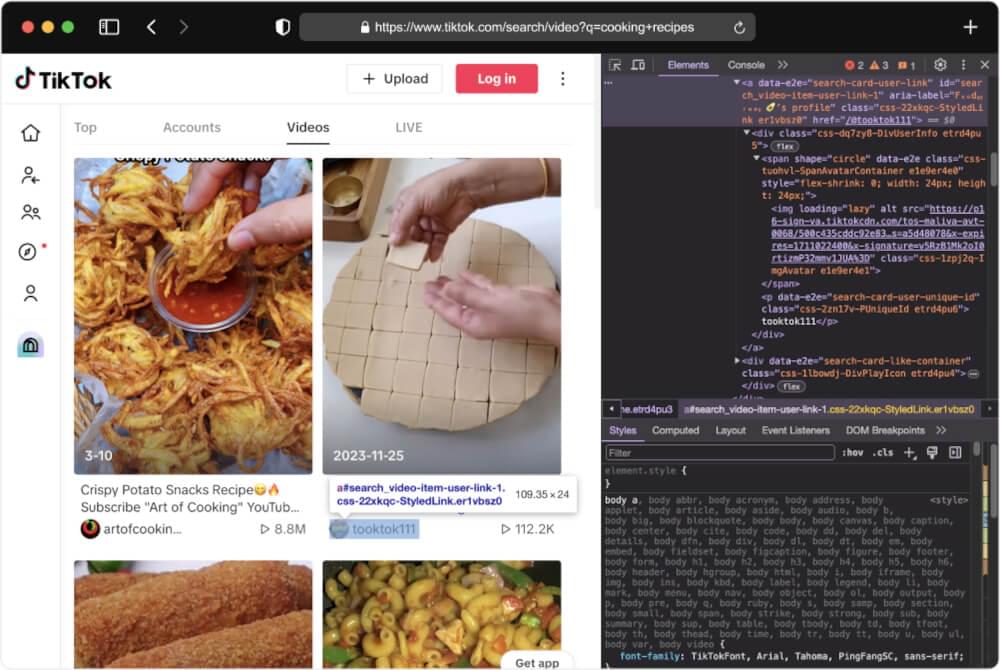
1 | # Function to scrape author information |
8. Scraping TikTok Video Hashtags
To scrape hashtags associated with TikTok videos in the search results, we’ll need to identify the HTML elements containing the hashtags and extract them accordingly.
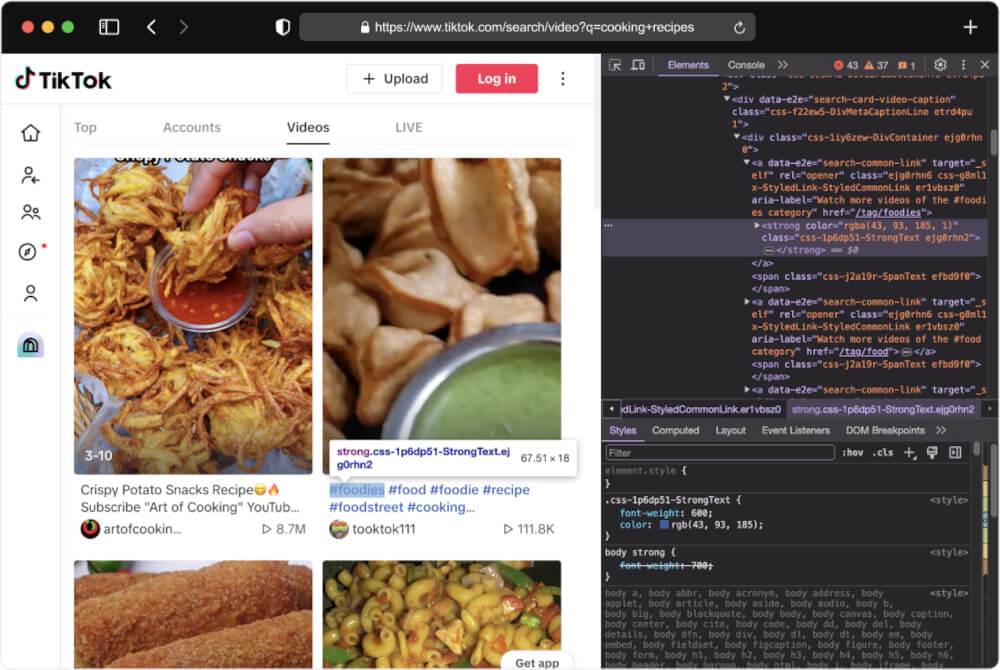
1 | # Function to scrape hashtags |
9. Complete Code - TikTok Scraper
Here’s the complete code integrating all the scraping tasks for scraping data from TikTok search results:
1 | from crawlbase import CrawlingAPI |
Example Output:
1 | [ |
10. Handling Pagination in TikTok scraper
When scraping TikTok data, it’s essential to navigate through multiple pages of search results efficiently. TikTok implements a scroll-based pagination system, where new content loads as the user scrolls down the page.
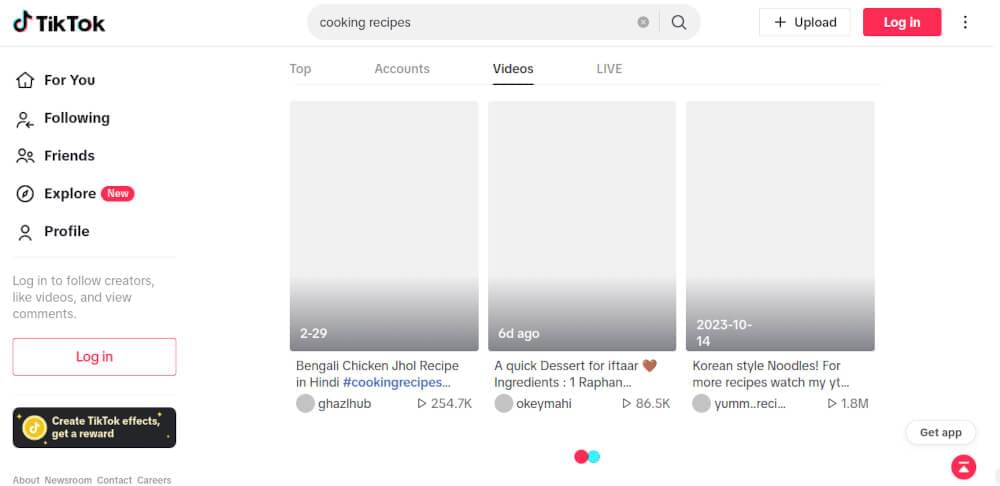
To handle this pagination, we can utilize the “scroll” parameter provided by the Crawlbase Crawling API. We’ll set the “scroll” parameter to “true” in our request to the Crawlbase Crawling API. This instructs the API to simulate scrolling down the page to load additional content. By default, the scroll interval is set to 10 seconds (10000 milliseconds). However, we can adjust this interval according to our requirements using the “scroll_interval” parameter.
We can update the options
object in our script to configure pagination handling as below:
1 | options = { |
11. Saving Scraped TikTok Data into a CSV File
Once we’ve successfully scraped TikTok data, it’s essential to save it for further analysis or usage. One common method for storing structured data is by saving it into a CSV file.
Here’s a function to save our scraped TikTok data into a CSV file:
1 | import csv |
You can call this save_to_csv
function with the scraped TikTok data and the desired filename (e.g. tiktok_data.csv) to save the data into a CSV file.
tiktok_data.csv
Snapshot:
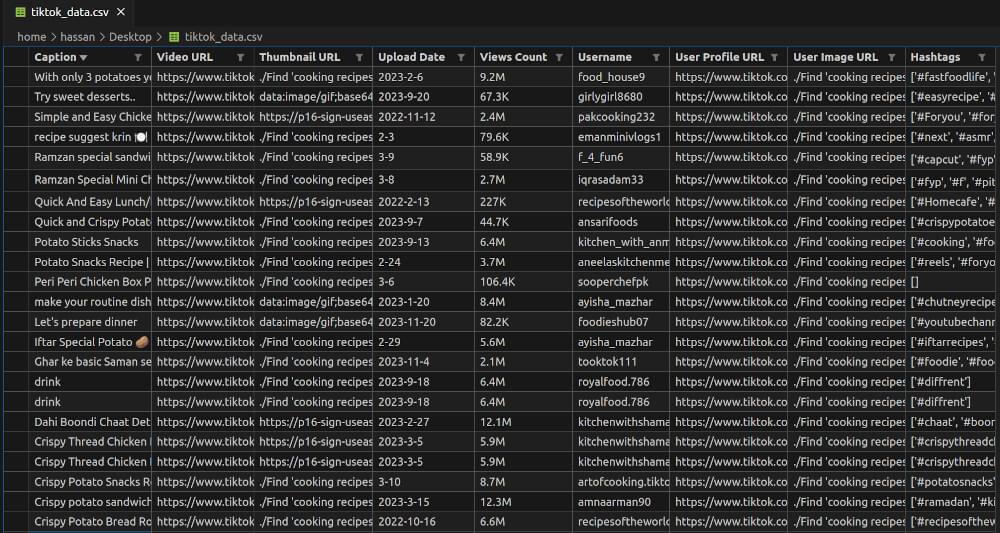
12. Final Thoughts
Congratulations on successfully creating your TikTok scraper using the Crawlbase Crawling API and Python! This guide has given you the know-how and tools to get important information from TikTok easily.
Also, have a look at the list of Tiktok scrapers we created for you.
Now that you’re good at TikTok scraping, you can do lots of things. You can get data from other social media accounts, do market research, follow trends, and more. And with the Crawlbase Crawling API, you can customize your scraping to fit exactly what you need.
If you’re looking to expand your web scraping capabilities, consider exploring our following guides on scraping other social media platforms.
For further customization options and advanced features, refer to the Crawlbase Crawling API documentation. If you have any questions or feedback, don’t hesitate to reach out to our support team. We’re here to assist you on your web scraping journey and help you achieve your data collection goals. Thank you for choosing the Crawlbase Crawling API, and we wish you success in all your scraping endeavors!
13. Frequently Asked Questions (FAQs)
Q. What is TikTok Scraping?
TikTok scraping involves extracting data from the TikTok platform, which includes information such as user profiles, video details, interactions, hashtags, and more. This process enables users to collect data for analysis, research, trend tracking, and other purposes.
Q. Why Scrape TikTok?
Scraping TikTok allows you to extract valuable data for various purposes, such as market research, trend analysis, content creation, and competitor analysis. By collecting information from TikTok, you can gain insights into user behavior, popular hashtags, trending topics, and engagement metrics. This data can be used to inform business decisions, improve marketing strategies, and identify opportunities for growth.
Q. Is It Legal to Scrape TikTok?
The legality of scraping TikTok depends on how you use the data and whether you comply with TikTok’s terms of service and data usage policies. While TikTok’s terms of service prohibit automated scraping of its platform, there may be cases where scraping is permissible for research, analysis, or personal use. However, it’s essential to review TikTok’s terms of service and consult with legal experts to ensure compliance with relevant laws and regulations.
Q. What Can You Scrape from TikTok?
You can scrape various types of data from TikTok, including user profiles, videos, comments, likes, shares, hashtags, and engagement metrics. With the right scraping techniques, you can extract information about trending content, popular creators, audience demographics, and user interactions. This data can provide valuable insights into TikTok’s ecosystem and help you better understand its audience and trends.
Q. What are the Best Ways to Scrape TikTok?
The best ways to scrape TikTok involve employing techniques tailored to overcome TikTok’s dynamic content loading and JavaScript rendering. Here are some recommended methods:
- Headless Browsers and Automation Tools: Utilize headless browsers or automation tools such as Selenium to simulate user interactions with TikTok’s website. By automating tasks like scrolling, clicking, and navigating, you can dynamically load content and extract data effectively, including JavaScript-rendered elements.
- TikTok Official APIs: TikTok’s official APIs offer a sanctioned way to access structured data, including user profiles, videos, comments, and likes. While they provide reliability and ease of use, they may have limitations on data access. Additionally, accessing TikTok’s official APIs may require registration and compliance with usage policies.
- Third-party API Providers: Consider using third-party APIs, like Crawlbase Crawling API, that offer TikTok data integration services. These providers offer comprehensive APIs with features tailored for data extraction, enabling seamless access to TikTok’s content without the need to handle complex scraping tasks.
By implementing these methods, you can effectively scrape TikTok for valuable insights, trends, and user-generated content while overcoming its unique challenges, including JavaScript rendering and anti-scraping measures.