Google is the most used search engine in the world, with over 8.5 billion searches a day. From businesses checking out competitors to researchers studying online trends, Google Search results are a treasure trove of data. By scraping this data you can extract titles, URLs, descriptions, and more and get actionable insights to make better decisions.
Scraping Google, however, is not easy. Its advanced anti-bot measures, frequent updates, JavaScript requirements, and legal considerations make it tough. But Crawlbase Crawling API has got you covered with its built-in Google SERP scraper that handles all these complexities for you.
In this post we will walk you through scraping Google Search results using Python and Crawlbase. Here’s what you will learn:
- Why you need to extract Google Search data.
- What data to extract, titles, links, and snippets.
- Challenges of scraping Google and how Crawlbase makes it easy.
- Using Crawlbase Crawling API to scrape Google SERPs.
Here’s a short video tutorial on how to scrape Google search results with Python:
Table of Contents
- Why Scrape Google Search Results?
- Key Data Points to Extract from Google Search Results
- Understanding the Challenges of Scraping Google
- Google’s Anti-Bot Measures
- Google SERP Latest JavaScript Requirement (2025)
- Crawlbase Built-in Google SERP Scraper
- Installing Python and Required Libraries
- Choosing the Right IDE for Scraping
- Writing Google SERP Scraper
- Handling Pagination
- Storing Scraped Data in a JSON File
- Complete Code Example
Why Scrape Google Search Results?
Google Search results are a goldmine of information that can power applications in SEO, competitor analysis, AI development, and more. Scraping this data will give you the insights to make better decisions and innovate.
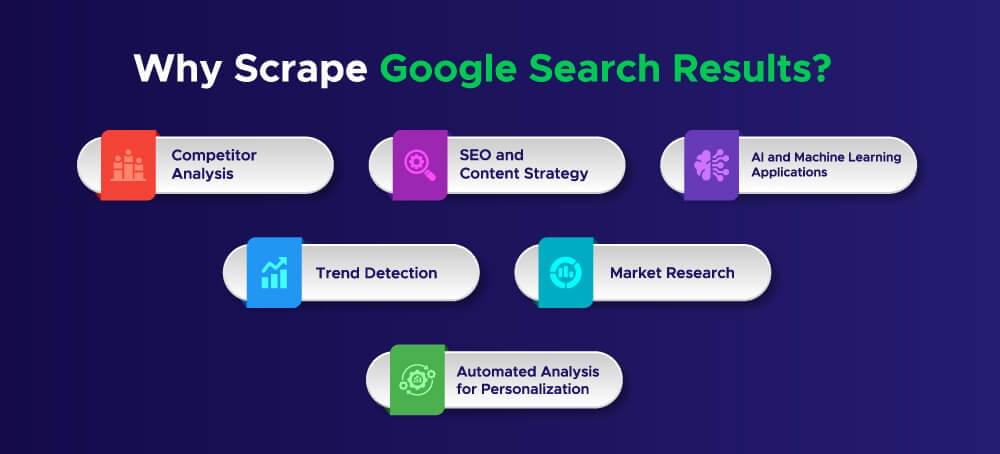
- Competitor Analysis: See competitor rankings, meta titles, descriptions, and strategies for specific keywords. Track the performance of a blog post in search results to monitor visibility and optimize content.
- SEO and Content Strategy: Find high-performing keywords, track website performance, and uncover content gaps to increase organic traffic. Scraping Google Search results supports search engine optimization by helping you track keyword rankings and optimize your content strategy.
- AI and Machine Learning: Train AI models for search optimization, NLP, and chatbots using real-world search queries and FAQs.
- Trend Detection: Stay ahead of industry trends by analyzing frequently searched terms and evolving user preferences.
- Market Research: Understand user intent, consumer behavior, and popular services directly from search results.
- Personalization and Automation: Use data to develop AI-driven personalization systems for targeted marketing or user-specific recommendations.
Scrape Google Search data to power your workflows and applications and get ahead of the competition across industries. Scraping allows you to extract search result data such as organic results, local results, related searches, related questions, and even Google Maps listings.
Key Data Points to Extract URLs from Google Search Results with Python
When scraping Google Search results you should be extracting relevant data. These key data points will help you to analyze trends, improve strategies or feed into AI models. Here’s what to look for:
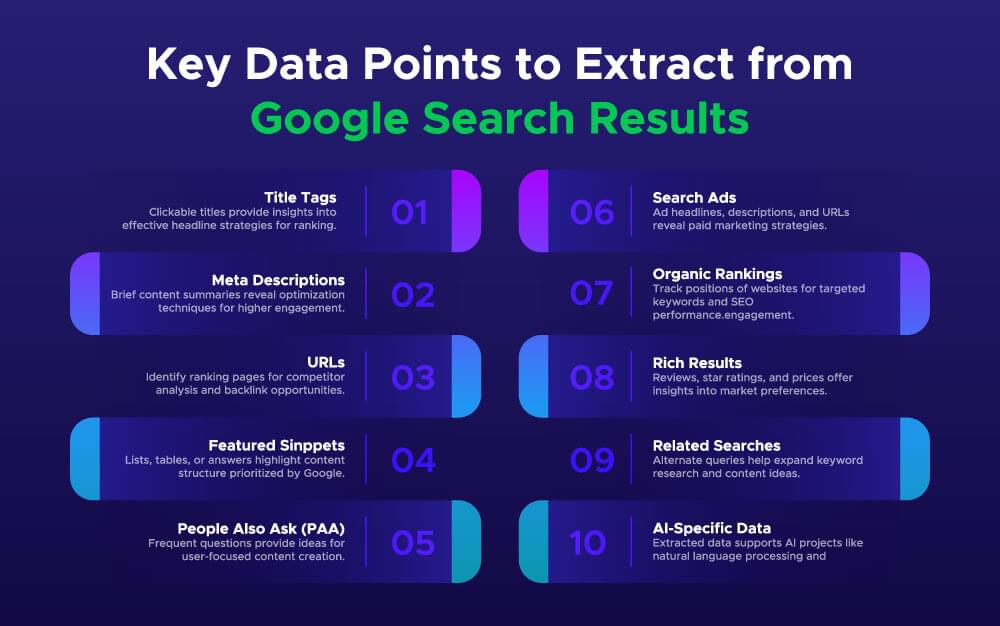
Understanding the Challenges of Scraping Google Search Results
Scraping Google Search results is more complicated than most websites because of Google’s anti-bot measures and technical requirements. Here’s a breakdown of the main challenges and how to tackle them responsibly:
Google’s Anti-Bot Measures
Google has systems in place to block automated bots. Here are some of the challenges:
- CAPTCHAs: Google displays CAPTCHAs to suspicious traffic and stops scraping until resolved.
- IP Blocking: Sending too many requests from the same IP address will get you temporarily or permanently blocked.
- Rate Limiting: Sending too many requests too quickly will trigger Google’s systems and flag your activity as suspicious.
Solution: To overcome these challenges, use the Crawlbase Crawling API with its built-in “google-serp” scraper. This scraper automatically rotates proxies, bypasses CAPTCHAs, and mimics human browsing behavior so you can get the data seamlessly.
Google SERP’s Latest JavaScript Requirement (2025)
As of 2025, Google search result pages (SERPs) will require JavaScript to be enabled in modern browsers for the search results to load. Without JavaScript, the page will not render, and users (and scrapers) will get an empty page.
Solution: Modern scraping tools like Crawlbase’s “google-serp” scraper handle JavaScript rendering so you can easily get fully rendered Google search results.
Crawlbase Crawling API for Google SERP Scraping
Crawlbase Crawling API is the best tool for scraping Google Search results. It handles JavaScript and anti-bot measures. With the built-in Google SERP scraper, you don’t need to configure anything.
Crawlbase Built-in Google SERP Scraper
Crawlbase has a built-in scraper for Google Search results called “google-serp“ scraper. This scraper handles JavaScript and bot protections automatically, so scraping is easy.
Benefits of Using Crawlbase Scrapers
- JavaScript Rendering: Handles JavaScript pages.
- Anti-Bot Bypass: Avoids CAPTCHAs and blocks.
- Pre-configured Google SERP Scraper: Scrapes with a ready-to-go scraper.
- IP Rotation & Error Handling: Reduces the risk of being blocked and ensures data collection.
With Crawlbase, scraping Google Search results is a breeze.
Setting Up Your Python Environment
Before you start scraping Google Search results, you’ll need to set up your Python environment. This section will walk you through installing Python, downloading the Crawlbase Python library, and choosing the best IDE for web scraping.
Getting Started with Crawlbase
- Sign Up for Crawlbase
To use the Crawlbase Crawling API, sign up on the Crawlbase website. After signing up, you’ll get your API tokens from the dashboard. - Obtain Your API Token
Once you’ve signed up, you will receive two types of API tokens: a Normal Token for static websites and a JS Token for JavaScript-heavy websites. For scraping Google Search results with the ‘google-serp’ scraper, you can use the Normal Token.
Installing Python and Required Libraries
If you don’t have Python installed, go to python.org and download the latest version for your operating system. Follow the installation instructions.
After installing Python, you need to install the Crawlbase library. Use the following commands to install Crawlbase:
1 | pip install crawlbase |
Choosing the Right IDE for Google SERP Scraping
For web scraping, choosing the right Integrated Development Environment (IDE) is important for your workflow. Here are some options:
- VS Code: Lightweight with many Python extensions.
- PyCharm: Feature-rich IDE with good support for Python and web scraping.
- Jupyter Notebook: Great for prototyping and data analysis in an interactive environment.
Pick one that’s fit you and you’re ready to start scraping Google Search results!
How to Scrape Google Search Results Using Python
In this section, we’ll show you how to build a Google search scraper, leveraging the Crawlbase Crawling API to handle JavaScript rendering and bypass anti-bot measures. We’ll also cover pagination and storing the scraped data in a JSON file.
Writing Google SERP Scraper
To scrape Google Search results, we will use the “google-serp” scraper provided by the Crawlbase Crawling API. This scraper handles all the heavy lifting, including rendering JavaScript and bypassing CAPTCHA challenges.
Here’s how to write a simple Google SERP scraper using Python:
1 | from crawlbase import CrawlingAPI |
The scrape_google_results
function takes a search query and a page number as inputs, constructs a Google search URL, and sends a request to the Crawlbase API using the built-in “google-serp” scraper. If the response is successful (status code 200), it parses and returns the search results in JSON format; otherwise, it prints an error message and returns an empty list.
Handling Pagination
Pagination is essential when scraping multiple pages of search results. Google paginates its results in sets of 10, so we need to iterate through pages by adjusting the start
parameter in the URL.
Here’s how you can handle pagination while scraping Google:
1 | def scrape_all_pages(query, max_pages): |
This function loops through pages starting from page 1 up to the max_pages
limit. If no results are returned, it stops the scraping process.
How to Store Scraped Data in a JSON File
Once you’ve gathered the data, you can store it in a structured JSON format for easy access and analysis. Below is a function that saves the scraped results to a .json
file.
1 | import json |
This function saves the scraped data to a file with the specified filename, ensuring the data is properly formatted.
Complete Code Example
Here’s the complete code that puts everything together:
1 | from crawlbase import CrawlingAPI |
Example Output:
1 | [ |
Final Thoughts
Scraping Google Search results using Python is good for SEO, market research, competitor analysis, and AI projects. With Crawlbase Crawling API you can bypass JavaScript rendering and anti-bot measures and make Google scraping easy and fast.
Using the built-in Crawlbase “google-serp” scraper, you can get search results without any configuration. This tool, along with its IP rotation and error-handling features, will make data extraction smooth.
Here are some other web scraping python guides you might want to look at:
📜 Scrape Yandex Search Results
📜 Scrape Yahoo Finance with Python
📜 How to Scrape Amazon
📜 How to scrape Walmart
📜 How to Scrape AliExpress
Sign up to start scraping today and unlock the potential of Google’s vast search data!
Frequently Asked Questions (FAQs)
Q. Is it legal to scrape Google Search results?
Scraping Google Search results can violate Google’s Terms of Service. However, using tools like Crawlbase Crawling API, you can follow best practices like limiting request frequency and not scraping personal data. Always follow ethical scraping guidelines and respect robots.txt files.
Q. Do I need to handle JavaScript when scraping Google?
Yes, Google Search results now require JavaScript to be enabled in your browser to load. Without it, you won’t see search results. Using Crawlbase Crawling API with its built-in Google SERP scraper, you can automatically handle JavaScript and get fully rendered results.
Q. How can I store scraped Google Search results?
You can store scraped data in a database, CSV / JSON file, or any other format that suits your needs. These formats allow you to store search results, including titles, URLs, and descriptions, for further analysis. You can follow the complete code example in this blog to save data in a JSON file efficiently.