Agoda has millions of hotel and property listings. Whether you’re doing research, building a travel aggregator, or analyzing hotel pricing, scraping data from Agoda can be really useful for your project.
In this guide, we’ll show you how to scrape hotel data from Agoda using Python and the Crawlbase Crawling API. You’ll learn how to extract hotel names, prices, reviews, and ratings while navigating Agoda’s scroll-based pagination. We’ll also cover how to set up your Python environment and store the scraped data in a structured format like JSON.
You’ll have an Agoda web scraper that can scrape dynamic content and fetch hotel listings. In addition to covering intermediate subjects like pagination and leveraging an API to facilitate scraping, this guide is designed with beginners in mind.
Ready to get started? Here’s what we’ll cover:
Table of Contents
- Why Scrape Hotel Data from Agoda?
- Key Data Points to Extract from Agoda
- Crawlbase Crawling API for Scraping Hotel Listings on Agoda
- Installing the Crawlbase Python Library
- Installing Python and Required Libraries
- IDE for Web Scraping
- Inspecting the HTML to Identify Selectors
- Writing the Agoda Search Listings Scraper
- Handling Scroll-Based Pagination
- Storing Scraped Data in a JSON File
- Complete Python Code Example
Why Scrape Hotel Data from Agoda?
Agoda is a popular online travel booking site trusted by millions of users worldwide for the best hotel deals. With millions of accommodations in its database, Agoda is a treasure trove of information for businesses, researchers, and developers. By scraping Agoda hotel data, you can get insights that are hard to get manually.
Here are some reasons why scraping Agoda hotel data is valuable:
1. Market Research
Scraping Agoda helps you to analyze hotel prices, trends, and availability for travel agencies, hotel managers, and competitors to optimize pricing and find opportunities.
2. Building a Travel Aggregator
Agoda data provides real-time hotel prices, ratings, reviews, and availability for users, for travel comparison sites or apps.
3. Competitor Analysis
Hotels can use Agoda’s data to track competitors’ pricing, promotions and reviews to improve pricing and services.
4. Personalized Recommendations
Scraping Agoda’s hotel data allows developers to create personalized travel recommendations based on amenities, ratings, and location.
5. Academic Research
Researchers can use Agoda’s data for studies on tourism trends, user behavior, and hospitality for academic projects and reports.
Key Data Points to Extract from Agoda
When scraping hotel data from Agoda, focusing on the most valuable data points will help you get the most out of your efforts. Here are the key data points to extract:
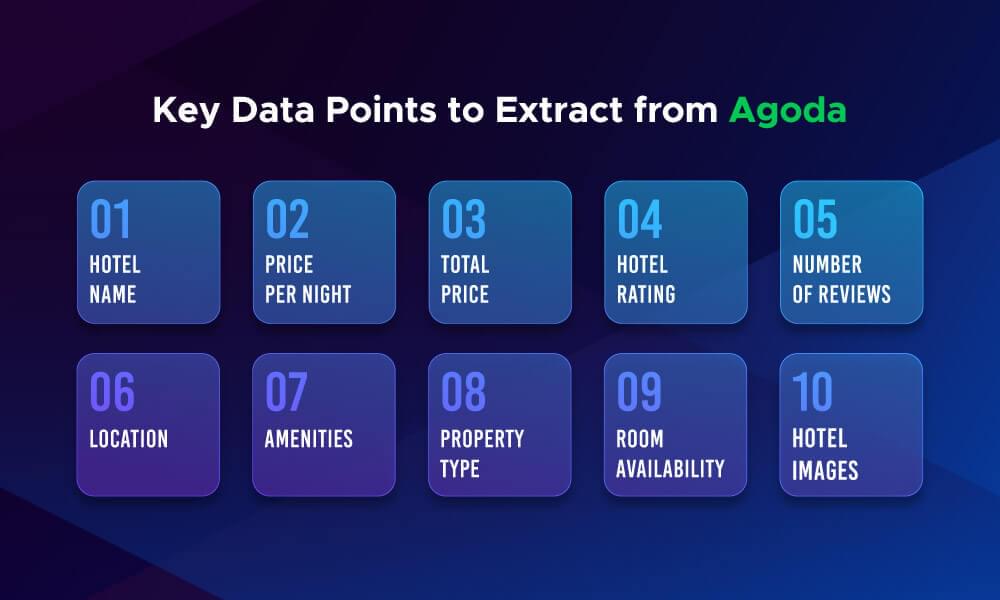
- Hotel Name – The name of the hotel helps identify properties.
- Price Per Night – The cost for a single night’s stay.
- Total Price – The full price for the entire stay, including taxes.
- Hotel Rating – Customer ratings to assess the quality of the property.
- Number of Reviews – Indicates the popularity and trustworthiness of the hotel.
- Location – City or neighborhood where the hotel is located.
- Amenities – Features like Wi-Fi, pool, gym, etc., that the hotel offers.
- Property Type – Type of accommodation, such as hotel, resort, or apartment.
- Room Availability – Information about available rooms during specific dates.
- Hotel Images – Visuals of the property are helpful for showcasing listings.
Crawlbase Crawling API for Scraping Hotel Listings on Agoda
Crawlbase Crawling API is the perfect tool for scraping Agoda, which has dynamic content that loads as you scroll. It handles JavaScript-rendered pages and bypasses security measures like IP blocks, so scraping is efficient and smooth.
Here’s why Crawlbase is suitable for Agoda scraping:
- Handles Dynamic Content: Crawlbase simulates human scrolling so all hotel listings are captured as the page loads more data.
- IP Rotation: Rotates IPs to avoid rate limits and blocks from Agoda.
- Fast and Reliable: Scrape lots of data quickly.
- Customizable Requests: Adjust headers, cookies, and request parameters to your needs.
Crawlbase Python Library
The Crawlbase Python library makes using the API easy. To get started, you’ll need your Crawlbase access token, which you can get by signing up for their service.
Here’s an example code to fetch data from Agoda using Crawlbase:
1 | from crawlbase import CrawlingAPI |
Note: A JS Token from Crawlbase is required to scrape JavaScript content. Crawlbase offers 1,000 requests free for its Crawling API. See the documentation for more. Next, we’ll set up your Python environment for Agoda scraping!
In the next section, we’ll set up your Python environment for Agoda scraping!
Setting Up Your Python Environment
Installing the libraries and configuring your environment are prerequisites for beginning Agoda scraping. Follow the below steps to complete the setup.
Installing Python and Required Libraries
Make sure you have Python installed on your computer. If not, download and install the latest version from the official Python website.
After installing Python, we need to install a few required libraries:
- Crawlbase Python Library: To interact with the Crawlbase Crawling API.
- BeautifulSoup: To parse HTML and extract data.
You can install these libraries using pip:
1 | pip install crawlbase beautifulsoup4 |
IDE for Web Scraping
An Integrated Development Environment (IDE) will make coding and running your scraper easier. Popular choices for Python are:
- VS Code: A lightweight editor with many Python extensions.
- PyCharm: A full-fledged IDE for Python.
- Jupyter Notebook: For interactive coding.
Pick one that suits you, and you’re good to go!
Scraping Agoda Property Listings
In this section, we will scrape Agoda property listings for the city “Kuala Lumpur”. The search URL is:
Agoda Search URL for Kuala Lumpur
We will go through the following steps to scrape the listings efficiently:
Inspecting the HTML to Identify Selectors
Before we start scraping, we need to understand the Agoda search results page’s HTML structure so we can determine the selectors for the hotel data we want to extract.
- Open the Agoda URL: Navigate to the Agoda search results page for Kuala Lumpur.
- Inspect the Page: Right-click on the page and choose “Inspect” or press
Ctrl + Shift + I
to open the Developer Tools.
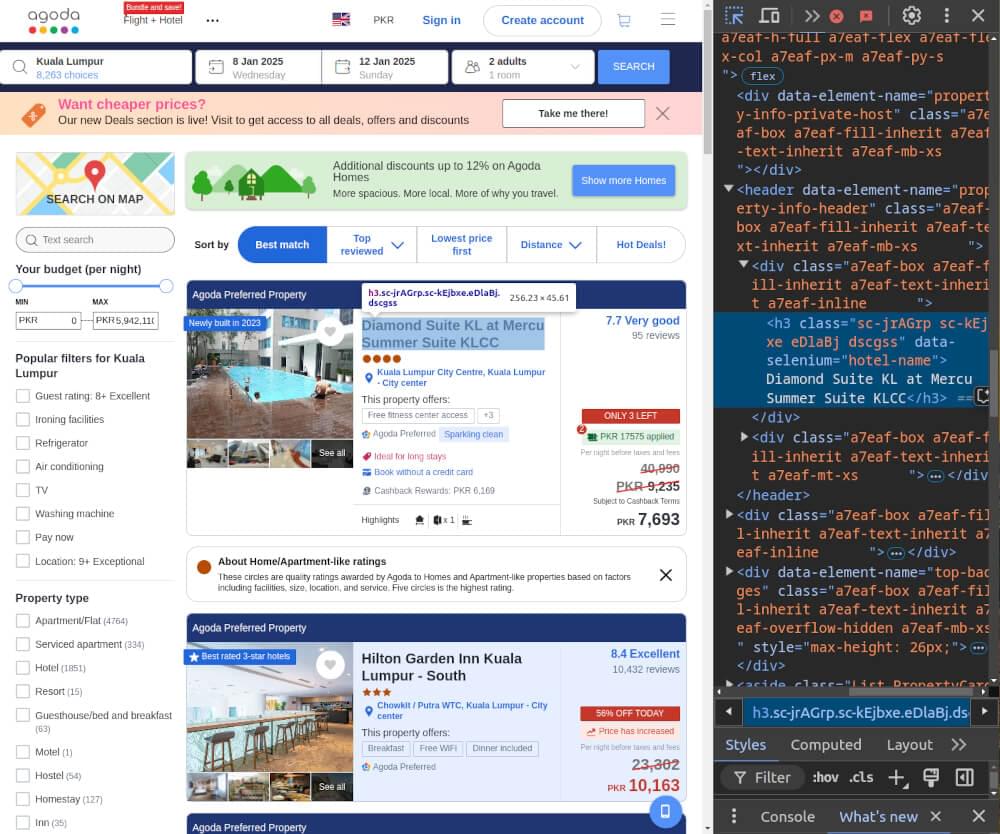
- Identify Key Elements: In the page’s HTML structure, find the elements that contain hotel details. We will focus on:
- Hotel Name: Within an
<h3>
element withdata-selenium="hotel-name"
. - Price: In a
<div>
element withdata-element-name="final-price"
. - Rating: In a
<p>
element withdata-element-name="review-score"
. - Hotel Link: In an
<a>
tag with classPropertyCard__Link
that links to the hotel’s page.
Writing the Agoda Search Listings Scraper
To scrape data from Agoda, we will use Crawlbase Crawling API to handle dynamic content and render the page like a real browser. This ensures that we capture all hotel listings, even those loaded by JavaScript.
Here is how we can write the scraper:
1 | from crawlbase import CrawlingAPI |
Handling Scroll-Based Pagination
Agoda uses scroll-based pagination, so more hotel listings will appear as you scroll down the page. We can instruct Crawlbase Crawling API to simulate scrolling and capture more listings.
To manage scrolling, we can use the scroll
and scroll_interval
options. Here’s how we can set them:
1 | options = { |
This will make the crawler scroll for 20 seconds, ensuring that all hotel listings are loaded before scraping.
Storing Scraped Data in a JSON File
Once we have the data we need to save it in a structured format like JSON so we can analyze or process the data later. Here’s how we can save the scraped hotel data to a JSON file:
1 | def save_to_json(data, filename='hotels_data.json'): |
Complete Python Code Example
Now that we have all the components in place let’s combine everything into a complete working example. This script will fetch the Agoda search results for Kuala Lumpur, extract hotel information, and store it in a JSON file.
1 | from crawlbase import CrawlingAPI |
Example Output:
1 | [ |
Final Thoughts
Scraping hotel data with Python and Crawlbase enables businesses to gain insights through competitive analysis, price monitoring, and market research. Using the Crawlbase Crawling API, you can scrape data from dynamic, JavaScript-heavy websites like Agoda without running into common issues like pagination or content loading delays.
In this blog, we covered everything from finding the key HTML elements on Agoda’s search results page to writing and running a complete Python scraper. We also showed how to handle scroll-based pagination and store the scraped data in a JSON file for further analysis.
If you’re interested in learning how to scrape data from other real estate websites, check out our helpful guides below.
📜 How to Scrape Realtor.com
📜 How to Scrape Zillow
📜 How to Scrape Airbnb
📜 How to Scrape Booking.com
📜 How to Scrape Expedia
If you have any questions or feedback, our support team is always available to assist you on your web scraping journey. Remember to follow ethical guidelines and respect the website’s terms of service. Happy scraping!
Frequently Asked Questions
Q. Is it legal to scrape hotel data from Agoda?
Web scraping is a gray area legally. While it’s generally acceptable to scrape publicly available data, always check Agoda’s terms of service to ensure compliance. Scrape responsibly and avoid using the data for unauthorized purposes.
Q. How do I handle CAPTCHA or anti-bot measures on Agoda?
Agoda uses CAPTCHAs and other bot-detecting techniques. You can get around these issues by using the Crawlbase Crawling API, which has features like browser-based rendering and IP rotation.
Q. Can I scrape data for multiple cities at once?
Yes, you can scrape data for multiple cities by modifying the query parameters in the Agoda URL. For example, update the city
parameter with the desired city’s ID. Just make sure to follow scraping best practices, like limiting request frequency, to avoid being blocked.