Using a proxy with cURL is a great way to manage web requests, hide your IP, and bypass network restrictions. Whether you’re web scraping, testing APIs or securing your internet traffic, proxies help by hiding your real IP and routing requests through a different server.
But first, what is cURL?
cURL (short for Client URL) is a command line tool to send and receive data using various network protocols like HTTP, HTTPS, FTP, and more. It’s a small but powerful tool used by developers to test, automate web requests, and fetch data from endpoints.
Here’s everything you need to know about using cURL with proxies. Installing cURL on different platforms, setting up HTTP, HTTPS, and SOCKS proxies, environment variables, persistent proxy settings, and how to bypass proxies. Let’s go!
Table of Contents
- For Windows
- For macOS
- For Linux
- Basic Syntax for HTTP Proxy
- Example of HTTPS Proxy
- Adding Authentication
- Handling SSL Certificate Errors
- Basic Syntax for SOCKS Proxy
- Authentication with SOCKS Proxy
- Using –socks Option
- For HTTP and HTTPS
- How to Unset Proxy Variables
- For Linux and macOS
- For Windows
- Ignoring Proxy for Specific Requests
- Quick Proxy Management Tips
- Final Thoughts
- Frequently Asked Questions
Why Use cURL With a Proxy?
Using a proxy with cURL is great for anyone working with web requests. A proxy is an intermediate layer between your computer and the internet, it sends your requests through its server. Here’s why proxies with cURL are so cool:
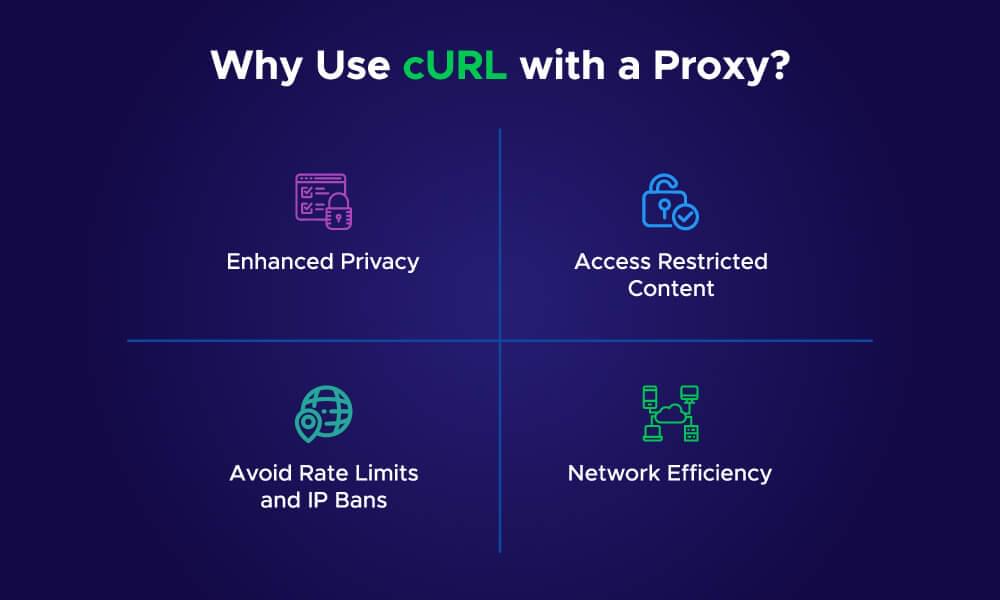
- Enhanced Privacy
Proxies hide your real IP address and replace it with theirs. So you stay anonymous while sending requests and safe online. - Access Restricted Content
Proxies help you avoid geographical restrictions or firewalls so you can access websites and data that would otherwise be blocked. - Avoid Rate Limits & IP Bans
When doing scraping or API testing, proxies allow you to rotate IPs so websites won’t block your requests. - Network Efficiency
Some proxy servers use caching which minimize the time needed to complete requests, overall performance will be better.
Whether you’re testing APIs, scraping web data or securely accessing the internet, using cURL with a proxy is a simple solution for your needs. In the next sections, we’ll show you how to set up and use cURL with different types of proxies.
Installing cURL on Different Platforms
Before you can use cURL with a proxy you need to make sure cURL is installed on your system. Here’s how to install cURL on Windows, macOS, and Linux.
For Windows
- Go to the cURL download page: curl.se/windows.
- Download the correct version for your system (32-bit or 64-bit).
- Extract the ZIP file.
- Add the curl.exe to your system’s PATH so you can use it from the command prompt.
To test the installation, run:
1 | curl --version |
For macOS
cURL is included with most macOS systems. To check if it’s installed, open the Terminal and type:
1 | curl --version |
If it’s not installed or you want the latest version, use Homebrew:
- Install Homebrew if it’s not already installed:
1 | /bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)" |
- Install cURL:
1 | brew install curl |
For Linux
Many Linux distributions include cURL by default. To check, open the terminal and run:
1 | curl --version |
If it’s not installed, you can easily install it depending on your distribution:
- Ubuntu/Debian:
1 | sudo apt update |
- CentOS/Fedora:
1 | sudo yum install curl |
Once installed, verify by running:
1 | curl --version |
Now that you have cURL installed, let’s move on to using cURL with HTTP and HTTPS proxies.
Using cURL With HTTP/HTTPS Proxy
Proxies are used to add privacy, bypass network restrictions, and scraping tasks. cURL makes it easy to work with HTTP and HTTPS proxies by allowing you to route your requests through a proxy server.
Basic Syntax for HTTP Proxy
To use an HTTP proxy with cURL you need to use the -x
or --proxy
option. Here’s the basic syntax:
1 | curl -x "http://proxy_address:port" "http://example.com" |
- Replace
proxy_address
with the proxy server’s address. - Replace
port
with the proxy’s port number. - Replace
http://example.com
with the URL you want to access.
Example:
If your proxy address is 127.0.0.1
and the port is 8080
, the command would look like this:
1 | curl -x "http://127.0.0.1:8080" "http://example.com" |
Example of HTTPS Proxy
When using HTTPS proxies, the process is similar. You need to specify the HTTPS protocol in the proxy address:
1 | curl --proxy "https://proxy_address:port" "https://example.com" |
Example:
Suppose your HTTPS proxy address is proxy.example.com
, and the port is 443
. The command would be:
1 | curl --proxy "https://proxy.example.com:443" "https://secure-site.com" |
Adding Authentication
If your proxy requires a username and password, you can include them in the command:
1 | curl -x "http://username:password@proxy_address:port" "http://example.com" |
Example:
For a proxy at 127.0.0.1:8080
with a username of user
and a password of pass
, the command would be:
1 | curl -x "http://user:[email protected]:8080" "http://example.com" |
Handling SSL Certificate Errors
When using HTTPS proxies, you might encounter SSL certificate errors. To bypass them (if you trust the proxy server) use the -k
option:
1 | curl --proxy "https://proxy.example.com:443" "https://secure-site.com" -k |
Using cURL with HTTP and HTTPS proxies hides your IP and allows you to access restricted websites. Next, we’ll use cURL with SOCKS proxies.
Using cURL With SOCKS Proxy
A SOCKS proxy is a lower-level proxy than HTTP and HTTPS proxies. It works with more types of traffic, including FTP and others. cURL supports SOCKS proxies so it’s great for web scraping or bypassing geo-restrictions.
Basic Syntax for SOCKS Proxy
To use a SOCKS proxy with cURL you can use the -x
or --proxy
option just like with HTTP/HTTPS proxies. The only difference is specifying the SOCKS protocol (SOCKS4 or SOCKS5). The basic syntax is:
1 | curl -x "socks5://proxy_address:port" "http://example.com" |
- Replace
proxy_address
with the SOCKS proxy server address. - Replace
port
with the proxy port number. - Replace
http://example.com
with the URL you want to visit.
Example:
If you are using a SOCKS5 proxy at 127.0.0.1
and the port is 1080
, the command will be:
1 | curl -x "socks5://127.0.0.1:1080" "http://example.com" |
Authentication with SOCKS Proxy
Some SOCKS proxies require authentication, just like HTTP/HTTPS proxies. To add a username and password for authentication, you can include them in the proxy URL:
1 | curl -x "socks5://username:password@proxy_address:port" "http://example.com" |
Example:
For a SOCKS5 proxy at 127.0.0.1
on port 1080
with username user and password pass, the command will be:
1 | curl -x "socks5://user:[email protected]:1080" "http://example.com" |
Using --socks
Option
In addition to the -x option, you can use --socks5
(or --socks4
for SOCKS4) to specify the proxy protocol explicitly. Here’s the syntax for SOCKS5:
1 | curl --socks5 "127.0.0.1:1080" "http://example.com" |
This approach is especially useful if you want to avoid confusion when working with multiple types of proxies.
Using SOCKS proxies with cURL is great when you need more flexibility and want to route different types of traffic, including FTP and others. In the next section, we’ll cover how to set up proxy settings using environment variables.
Setting Proxy Using Environment Variables
You can set a proxy for cURL using environment variables, which is useful for applying the same proxy to multiple cURL commands.
For HTTP and HTTPS
To set the proxy for HTTP and HTTPS requests, use these commands in your terminal:
1 | export http_proxy="http://user:password@proxy_address:port" |
- Replace
user:password
with your proxy credentials. - Replace
proxy_address
with the IP or domain of the proxy server. - Replace
port
with the proxy’s port number.
Example:
1 | export http_proxy="http://user:[email protected]:1080" |
How to Unset Proxy Variables
To stop using the proxy, run these commands:
1 | unset http_proxy |
This will remove the proxy settings for the current session.
In the next section, we’ll cover how to configure cURL to always use a proxy.
Configuring cURL to Always Use a Proxy
If you want cURL to always use a proxy without needing to set it every time, you can configure it to do so by editing a configuration file.
For Linux and macOS
- Open the terminal and go to your home directory:
1 | cd ~ |
- Check if you have a
.curlrc
file. If not, create one:
1 | nano .curlrc |
- Add your proxy settings inside this file:
1 | proxy="http://user:password@proxy_address:port" |
- Save the file and exit. Now, cURL will use the proxy settings automatically for every request.
For Windows
On Windows, the configuration file is named _curlrc
and is located in the %APPDATA%
directory.
- Open the command prompt and run this command to find the path:
1 | echo %APPDATA% |
Go to the directory path shown and create a file named
_curlrc
.Inside this file, add your proxy settings:
1 | proxy="http://user:password@proxy_address:port" |
- Save the file, and cURL will use the proxy for all future requests.
This method helps streamline your cURL usage and ensures your proxy is always set. In the next section, we’ll discuss how to ignore the proxy for specific requests.
Ignoring Proxy for Specific Requests
There may be times when you want to bypass the proxy for certain requests while using cURL. Fortunately, cURL offers an easy way to do this.
How to Ignore the Proxy
To bypass the proxy for a specific request, use the --noproxy
option. This tells cURL not to use the proxy for the URL you specify. Here’s how it works:
1 | curl --noproxy "*" "http://example.com" |
In this example, the *
wildcard ensures that no proxy will be used for http://example.com
. This is useful when you want to make direct connections to specific websites while keeping the proxy settings active for others.
Ignoring Proxy for Specific Domains
You can also ignore the proxy for specific domains or IP addresses. For instance, if you want to avoid using the proxy for domain example.com
, you can set the --noproxy
option like this:
1 | curl --noproxy "example.com" "http://example.com" |
This will not use the proxy for domain example.com
but will use the proxy for other requests.
Using the --noproxy
flag you can control when to use the proxy and when to connect directly to a website. This is important for managing your network connections.
In the next section, we’ll go over some quick tips for proxies.
Quick Proxy Management Tips
Managing proxies can save you time and effort if you switch between proxy configurations often. Here are some quick tips to help you manage proxies with cURL
1. Turn Proxy On and Off Quickly
If you switch between using a proxy and not using one often you can create aliases in your shell configuration file. This way you can turn the proxy on and off with a single command. For example, you can add the following aliases to your .bashrc
or .zshrc
file:
1 | alias proxyon="export http_proxy='http://user:[email protected]:1234'; export https_proxy='http://user:[email protected]:1234'" |
After saving the file, run:
1 | source ~/.bashrc # or source ~/.zshrc |
Now, you can quickly turn the proxy on by typing proxyon and turn it off by typing proxyoff. This makes it easy to switch proxy settings as needed.
2. Use a Proxy for Specific URLs Only
If you want to use the proxy only for certain URLs, set the environment variables temporarily for that specific session.
1 | export http_proxy="http://user:[email protected]:1234" |
This command ensures that the proxy is used only for domain example.com
. When you no longer need the proxy, simply unset the variables:
1 | unset http_proxy |
3. Test Proxy Connection
It’s essential to test your proxy settings before using them in production. You can do this by running a simple cURL command to check the IP address:
1 | curl "https://httpbin.org/ip" |
If everything is set up correctly, you should see the IP address of the proxy server instead of your own.
Final Thoughts
Using a proxy with cURL is a powerful way to hide your privacy, manage traffic, and bypass restrictions. Whether you’re working with HTTP, HTTPS or SOCKS proxies, cURL makes it easy to route your requests through different servers. With the ability to set proxies using command line options, environment variable, or configuration files, you have full control over how your requests are handled.
Follow this guide and you’ll be able to use cURL with proxies in your workflow in no time, perfect for web scraping, automation or even just simple API requests. Proxies help hide your IP and avoid geographical restrictions so are essential for many users who need secure and anonymous browsing. If you’re looking for a proxy provider, you can choose Crawlbase’s Smart Proxy service, one of the best in the market.
Regardless of Linux, macOS, or Windows, the process is the same, and with the right setup you can manage your proxies. Always test your configuration regularly to make sure everything is working smoothly. Happy coding!
Frequently Asked Questions
Q. What is a proxy in cURL?
A proxy in cURL is an intermediate server that sits between your computer and the internet. Using a proxy, cURL routes your requests through the proxy server instead of going directly to the website. This hides your real IP, improves privacy, and bypasses geographical restrictions.
Q. Can I use cURL with a SOCKS proxy?
Yes, you can use cURL with SOCKS proxies, like SOCKS4 or SOCKS5. Just add the right protocol to your cURL command, like socks5://
for SOCKS5 proxies. This is helpful when working with network configurations that need SOCKS or while preserving anonymity.
Q. How do I set a default proxy for cURL?
You can set a default proxy for cURL using a configuration file. For Linux and macOS, create a .curlrc
file in your home directory where you can put your proxy details. For Windows, it’s _curlrc
in the %APPDATA%
directory. This way cURL will always use the proxy without you needing to specify it in each command.