When working with websites or APIs, HTTP headers are key. They carry extra info between your device and the server, like what kind of data you’re sending or requesting, auth tokens, or language preferences. These headers help the website understand your request and respond correctly.
cURL is a command line tool that makes sending and customizing headers in your requests a breeze. Whether you’re testing an API, debugging an app, or scraping data, knowing how to send headers with cURL is a skill to have. For more advanced scraping projects where header management becomes complex, Crawlbase’s Crawling API handles these technical details automatically, helping you avoid blocks and CAPTCHAs without manual header configuration.
In this blog, we’ll go through how to send headers with cURL. You’ll learn the basic syntax, how to add one or multiple headers, and how to view HTTP response headers. If you find yourself needing a more robust solution for your production scraping needs, you’ll discover how our tools can save you hours of troubleshooting header-related issues. Let’s get started!
Table of Contents
- Why Use HTTP Headers With cURL?
- Basic Syntax for Sending HTTP Headers
- Sending a Custom HTTP Header With cURL
- Sending Multiple HTTP Headers in a Single Request
- Viewing HTTP Response Headers
- Removing or Overriding Default Headers
- Final Thoughts
- Frequently Asked Questions
Why Use HTTP Headers With cURL?
HTTP Headers are important when dealing with websites or APIs. You can pass extra information with your requests so the server can understand and process them better. By sending the correct headers, you can customize your communication with a server, access restricted resources, or request data in a specific format.
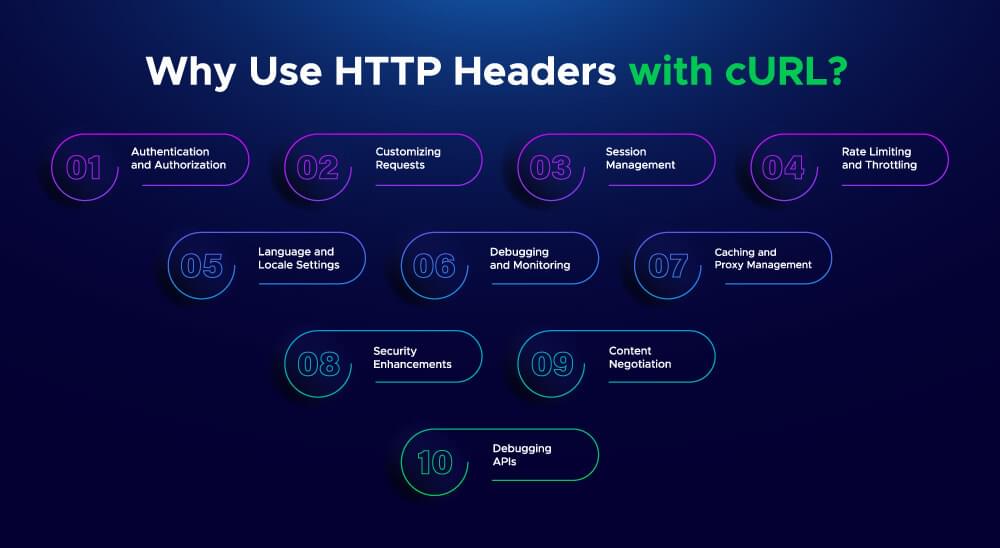
cURL with headers gives you more control over your requests. Whether you need to authenticate with an API, set a User-Agent
, or request JSON responses, headers are the way.
Understanding how to use HTTP headers with cURL ensures your requests are accurate and effective, whether you’re debugging, testing APIs, or working on a web scraping project.
Next, we’ll look at how to send and manage HTTP headers with cURL.
Basic Syntax for Sending HTTP Headers
When using cURL to send HTTP headers, you’ll often use the -H
or --header
option. This option allows you to specify a custom header in your HTTP request. The format is simple:
1 | curl -H "Header-Name: value" URL |
Here’s how the syntax breaks down:
-H
or--header
: Indicates you’re sending an HTTP header."Header-Name: value"
: The header you want to send. The header name is followed by a colon and its value.URL
: The address of the website or API you’re requesting.
Example: Sending a User-Agent Header
The User-Agent header tells the server about the client making the request. cURL includes a User-Agent by default, but you can customize it:
1 | curl -H "User-Agent: MyCustomUserAgent" https://example.com |
In this example, the server will see MyCustomUserAgent instead of the default cURL identifier.
Example: Sending an Authorization Header
If you’re accessing a protected resource, you may need an Authorization header to provide credentials. Here’s how to send a token-based authentication header:
1 | curl -H "Authorization: Bearer your-access-token" https://api.example.com/data |
This sends your API token in the request, giving you access to secured endpoints.
Now that you know the basics of the syntax, you can send custom HTTP headers with cURL to talk to servers and APIs more. Next up, we’ll get into sending multiple headers, default headers, and more.
Sending a Custom HTTP Header With cURL
cURL’s most powerful feature is the ability to send custom headers. This is super useful when you are working with APIs or websites that require specific headers to process requests.
To send a custom header with cURL, use -H followed by the header name and value in quotes.
Example: Sending a Custom Content-Type Header
The Content-Type
header tells the server what kind of data you’re sending in your request. For example, if you’re sending JSON to an API, you might specify the Content-Type
like this:
1 | curl -H "Content-Type: application/json" https://api.example.com/endpoint |
This ensures the server knows to parse your request as JSON.
Example: Custom Headers for Debugging
Custom headers can also be useful for testing and debugging. Let’s say you want to add an X-Debug
header to track your request during development:
1 | curl -H "X-Debug: true" https://example.com |
In this case, the server might log your request differently to help you debug.
Custom headers are important when dealing with APIs or testing server behavior. They allow you to modify how your request is processed, making cURL a very useful tool for developers and analysts.
Next, we’ll look at how to send multiple headers in one cURL request.
Sending Multiple HTTP Headers in a Single Request
Sometimes, you may need to send multiple HTTP headers in a single cURL request. For example, APIs often require headers like Authorization
, Content-Type
, or custom headers for specific features. With cURL, you can add multiple headers by using the -H
option for each header.
Example: Sending Multiple Headers
Here’s how you can send multiple headers in one request:
1 | curl -H "Authorization: Bearer your-access-token" -H "Content-Type: application/json" https://api.example.com/endpoint |
In this example:
- The
Authorization
header is used to pass an access token during authentication. - The
Content-Type
header indicates that the request body is in JSON format.
Key Tip: Header Order
cURL processes headers in the order you specify them. If you override a header later, the new value will overwrite the previous one. Sending multiple headers allows you to customize your HTTP requests to exactly what the API or server needs. Next, we’ll look at how to view HTTP response headers with cURL.
Viewing HTTP Response Headers
When working with HTTP requests, you often want to check the response headers the server returns. Response headers contain important information like status codes, content types, and caching policies. cURL has simple options to view these headers.
Use -I
or --head
to Fetch Only Headers
The -I or –head option allows you to fetch only the response headers without downloading the body content. Here’s an example:
1 | curl -I https://example.com |
This command retrieves and displays just the response headers from the specified URL.
Use -i
or --include
to Show Headers With Body
If you want to view both the response headers and the content, use the -i or –include option:
1 | curl -i https://example.com |
This command includes the response headers along with the response body in the output.
Example Output
Here’s a sample of what response headers might look like:
1 | HTTP/1.1 200 OK |
Why Check Response Headers?
- Debugging: Identify server issues or misconfigured headers.
- Performance: Inspect caching policies or compression settings.
- Data Handling: Make sure the right content type is returned.
Checking response headers helps you see how the server is talking, and your requests are working. Now, let’s look at how to remove or override default headers in cURL.
Removing or Overriding Default Headers
cURL adds some default headers like User-Agent
or Accept
. But there are cases where you need to remove or override these headers to customize your requests or meet specific API requirements.
Removing Default Headers
To remove a default header, type the header name followed by a colon (:
) with no value. For example, to remove the User-Agent
header:
1 | curl -H "User-Agent:" https://example.com |
This command ensures that the User-Agent
header is not included in the request.
Overriding Default Headers
To override a default header, use the -H
option with the new value for the header. For example, to modify the User-Agent
header:
1 | curl -H "User-Agent: MyCustomUserAgent" https://example.com |
In this case, cURL replaces the default User-Agent
value with your custom value.
Practical Example
Here’s an example of overriding one header and removing another in the same request:
1 | curl -H "User-Agent: MyCustomUserAgent" -H "Accept:" https://example.com |
This command sets a custom User-Agent and removes the Accept header.
Why Remove or Override Headers?
- Avoid Detection: Customizing User-Agent to get past website blocks that block default cURL headers.
- API Compatibility: Some APIs require specific headers or block default ones.
- Cleaner Requests: Fewer headers means less complexity.
Customizing your headers gives you more control over how your requests interact with servers, improving flexibility and precision.
Final Thoughts
Sending HTTP headers with cURL is key to API interaction and web scraping. Customizing headers allows you to authenticate requests, fetch data in specific formats, and talk to servers in the right way.
With cURL’s simple commands, you can send custom headers, handle multiple headers, and override defaults with ease. Learn these, and you’ll be able to speed up your workflows and meet API or website requirements.
However, for production-level scraping where you need reliability at scale, consider Crawlbase’s suite of tools. Our Crawling API and Smart Proxy solutions handle complex header management automatically, helping you bypass CAPTCHAs and avoid IP blocks that often plague manual cURL approaches. When your projects move beyond testing to real-world data collection, sign up for Crawlbase to save countless hours troubleshooting failed requests and focus on what matters - the data itself.
Get started today and get more out of cURL, or take your web scraping to the next level with a Crawlbase solution tailored to your needs.
Frequently Asked Questions
Q. How can I add custom HTTP headers using cURL?
To include custom HTTP headers in cURL, use the -H option followed by the header name and value in the following format:
1 | curl -H "Header-Name: value" https://example.com |
For example, to add a custom User-Agent:
1 | curl -H "User-Agent: MyCustomAgent" https://example.com |
Q. How can I send multiple HTTP headers in one cURL request?
You can send multiple headers by using the -H option multiple times in the same command. Each -H should specify a different header:
1 | curl -H "Header-One: value1" -H "Header-Two: value2" https://example.com |
This helps include authentication tokens, content types, and other metadata in the same request.
Q. How do I view HTTP response headers with cURL?
To view only the response headers, use the -I or –head option:
1 | curl -I https://example.com |
If you want to include the headers along with the content, use the -i option:
1 | curl -i https://example.com |
This is useful for debugging or inspecting server responses.