Web scraping is a necessary means of extracting information off the web pages. BeautifulSoup is an effective and quite friendly Python package that generally makes collecting data for research, analysis, and automating repetitive tasks easy. The steps for utilizing BeautifulSoup to scrape data from the web will be discussed in this blog.
BeautifulSoup is actively and widely used all over the world for XML and HTML page conversion into Python objects. Novice programmers can easily use it because the package has a simple interface for locating and collecting the required dimensions.
New to web scraping and Python, or want to brush up on your skills? After reading this blog, you will know how to work with BeautifulSoup.
Here’s a short BeautifulSoup web scraping tutorial:
Table of Contents
- Installing Required Libraries
- Creating Your Project
- What is the DOM?
- How BeautifulSoup Interacts with HTML
- Parsing HTML
- Extracting Data with
find()
andfind_all()
- Navigating Tags and Attributes
- Step-by-Step Script Example
- Scraping Data from a Website
- Handling Errors
- Managing Dynamic Content
- Handling Pagination
- Avoiding Getting Blocked
- Respecting Website Terms and Conditions
- Avoiding Overloading Servers
Why Use BeautifulSoup in Python?
BeautifulSoup is one of the best-known Python libraries for web scraping in Python for its simplicity and efficacy. It allows you to pull out information from websites by means of HTML and XML documents.
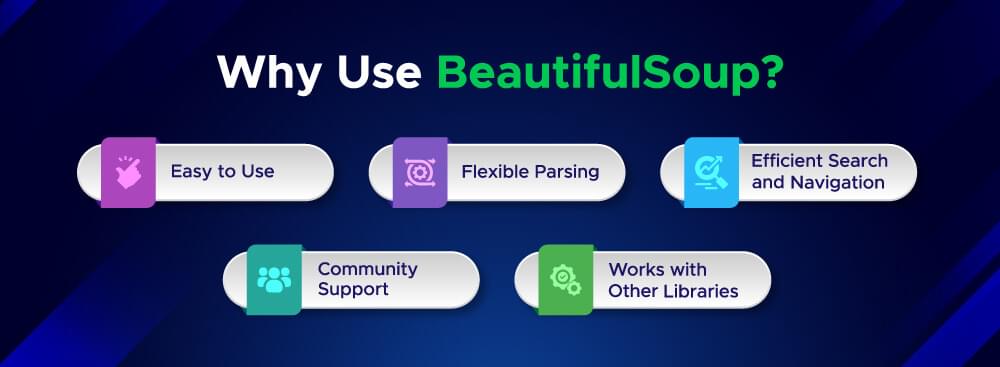
Easy to Use
BeautifulSoup is easy to use and you can scrape websites in just a few lines of code, perfect for beginners.
Flexible Parsing
It supports multiple parsers like the default Python HTML parser, lxml, and html5lib, so it’s adaptable to any web structure.
Efficient Search and Navigation
BeautifulSoup allows you to search and navigate through HTML elements. With find() and find_all(), you can extract data like text, links, or images.
Community Support
Big community, so you’ll find many tutorials and answers to common questions.
Works with Other Libraries
BeautifulSoup can be easily used with Selenium for dynamic content and Requests to retrieve websites.
In short, BeautifulSoup is a reliable, flexible, and easy-to-use tool for web scraping, suitable for beginners and experts alike.
Setting Up Your Environment
Before you start scraping websites with BeautifulSoup, you need to set up your development environment. This means installing the required libraries and creating a project directory for your scripts.
Installing Required Libraries
You’ll need two main libraries: Requests and BeautifulSoup4.
- Requests for fetching web pages.
- BeautifulSoup4 for parsing the HTML content of the web page.
Run the following commands in your terminal or command prompt to install these libraries:
1 | pip install requests |
These will allow you to send HTTP requests to websites and parse the HTML content for data extraction.
Creating Your Project
Now that you have the libraries installed, it’s time to set up your project. Create a new directory where you’ll put your Python scripts. For example, create a folder called beautifulsoup_scraping:
1 | mkdir beautifulsoup_scraping |
This will keep your web scraping project tidy and ready to go. Now, you’re ready to start scraping with BeautifulSoup.
Understanding HTML and the DOM
Before you start web scraping with BeautifulSoup, you need to understand the structure of web pages. Web pages are built with HTML (Hypertext Markup Language) and styled with CSS. The DOM (Document Object Model) is the structure of a web page as a tree of objects, which makes it easier to navigate and extract information from.
What is the DOM?
The DOM is a tree of an HTML document. It nests the content. Each node in the tree is an element, which can be a tag (like <p>
, <div>
, <a>
) or content (text within those tags). The DOM structure is what web scraping tools like BeautifulSoup work with to extract data from a web page.
For example, in a simple HTML document, you might have:
1 | <html> |
In this case, the DOM would have nodes for the <html>
, <body>
, <h1>
, <p>
, and <a>
elements, each containing their corresponding content.
How BeautifulSoup Interacts with HTML
BeautifulSoup uses the DOM to parse the HTML and create a tree of Python objects. So you can navigate through the structure and get the data you need. BeautifulSoup will automatically select the best parser available on your system so you can start right away.
When you load an HTML into BeautifulSoup, it becomes a tree of objects. Then, you can use various methods to find elements or tags, extract content, and manipulate the data.
For example, to find all the
tags (paragraphs) in the document, you can use:
1 | soup.find_all('p') |
This will help you focus on specific parts of the web page so scraping becomes more efficient and targeted.
By knowing HTML and the DOM, you can navigate web pages better and scrape only what you need.
Using BeautifulSoup for Web Scraping
Now that you have a basic understanding of HTML and the DOM, it’s time to start scraping data using BeautifulSoup. BeautifulSoup makes it easy to extract information from web pages by parsing HTML or XML documents and turning them into Python objects.
Parsing HTML
First, you need to load the web page content. You can use requests to fetch the HTML of a web page. Once you have the HTML, BeautifulSoup will take over and parse it for you.
Here’s how you can load and parse HTML using BeautifulSoup:
1 | from bs4 import BeautifulSoup |
After this, you can start navigating and extracting data from the page using BeautifulSoup’s powerful functions.
Extracting Data with find()
and find_all()
BeautifulSoup provides several methods to search for and extract elements from the page. The two most commonly used methods are find()
and find_all()
.
find()
: This method searches the document and returns the first match that fits the search criteria. It’s useful when you know there is only one element you want to extract.
1 | title = soup.find('h1') # Finds the first <h1> tag |
find_all()
: This method returns all matching elements as a list. It’s useful when you want to extract multiple elements, such as all the links or all the paragraphs on a page.
1 | paragraphs = soup.find_all('p') # Finds all <p> tags |
Both methods can also use attributes to narrow down the search. For example, you can search for a specific class or ID within a tag.
1 | # Finding a specific class |
Navigating Tags and Attributes
BeautifulSoup allows you to not only search for tags but also navigate through them and access specific attributes. Every HTML element has attributes that provide additional information, such as href
for links, src
for images, and alt
for image descriptions.
To access an attribute, use the ['attribute_name']
syntax. For example:
1 | # Get the href attribute of the first link |
You can also use nested searches to find tags inside other tags. This is useful when you need to get inside containers like divs or lists.
1 | # Find all <p> tags within a specific <div> |
With these tools, you can get and manipulate data from any webpage. The flexibility and simplicity of BeautifulSoup make it perfect for web scraping.
5. Creating Your First Web Scraping Script
Now that you know how to use BeautifulSoup for parsing and getting data, let’s put it into practice. In this section we will build a full web scraping script step by step.
Step-by-Step Script Example
Let’s go through the process of creating a simple web scraping script to get data from a webpage.
- Import Libraries: You need to import
requests
to get the webpage and BeautifulSoup to parse its HTML. - Get the Web Page: Use
requests
to get the HTTP GET request to the website. - Parse the HTML: Use
BeautifulSoup
to parse the HTML. - Extract Desired Data: Use
find()
orfind_all()
to extract text, links, or images.
Here’s a complete example:
1 | import requests |
Scraping Data from a Website
Let’s look at the script above:
- Target URL: We’re using
http://quotes.toscrape.com
, which has some example data to scrape. - Fetch the Page:
requests.get()
gets the HTML of the page. We checked the response code to see if the request was successful. - Parse with BeautifulSoup: BeautifulSoup parses the HTML text into a parse tree.
- Extract Data:
find_all()
finds all<span>
tags with class text to get the quotes.find_all()
finds all<small>
tags with the classauthor
to get the author’s names.
- Print the Results: The
for
loop iterates over the quotes and authors and prints them.
Running the Script
Save the script as scraper.py
and run with the following command:
1 | python scraper.py |
Expected Output:
1 | Quote: "The world as we have created it is a process of our thinking. It cannot be changed without changing our thinking." |
This script is a good starting point for any BeautifulSoup web scraping project. From here, you can add more functionality, like handling pagination, saving the data to a file, or scraping more complex websites.
By following this, you can extract data from any web page using BeautifulSoup and Python.
Handling Common Issues in Web Scraping
When web scraping, one is likely to come across issues. Sites may not work correctly; pages may be loaded with the help of JavaScript; data may be located on different pages. In this section, we will see how to handle these utilizing BeautifulSoup and other tools.
1. Handling Errors
Errors are everywhere in web scraping but can be handled:
- HTTP Errors: Every time a page is inaccessible due to some form of Error, it returns an HTTP status code like 404 (Not Found) or 500 (Server Error). The script should use error prevention techniques to not render any status code other than 200 as an issue.
Example:
1 | response = requests.get('http://example.com') |
- Missing Elements: Sometimes, the elements you want to scrape are not present on the page. So it’s good to put a condition to check if these elements are missing from the output before extracting any data.
Example:
1 | element = soup.find('div', class_='data') |
2. Managing Dynamic Content
Some websites load content via JavaScript after the page loads. In this case, the static HTML you scrape may not have the data you want.
Solution: Use Selenium or Playwright as they are browser automation tools that can load dynamic content.
Example with Selenium:
1 | from selenium import webdriver |
This allows you to interact with dynamic pages just like a regular user.
3. Handling Pagination
Websites split data across multiple pages, like blog posts or product listings. To extract all content from a website, you need to handle pagination by going through each page.
Solution: Find the next page link and loop through it until you reach the end.
Example:
1 | import requests |
In this script:
- The
while
loop goes through each page and extracts the quotes. - The
next
link is detected dynamically and appended to the base URL.
4. Avoiding Getting Blocked
Scraping a site too aggressively will get you blocked. Here’s how to avoid that:
- Add Delays: Use
time.sleep()
to pause between requests. - Rotate User-Agents: Send requests with different user-agent headers to mimic real browsers.
- Use Proxies: Route requests through multiple IP addresses using proxy servers. Crawlbase also has a Smart Proxy service that is super fast, easy to integrate, and affordable with a pay-as-you-go pricing model.
By addressing these common web scraping challenges, you’ll make your BeautifulSoup scripts more robust and reliable. Whether it’s handling errors, managing dynamic content, or avoiding rate limits, these tips will get your scraping projects running smoothly.
Ethical Web Scraping Practices
Web scraping should be done responsibly so you don’t harm websites and their servers. Here are the ethical practices to follow:
1. Respecting Website Terms and Conditions
Always check the Terms and Conditions or robots.txt file before scraping a site. This file tells you what can and can’t be scraped.
- Check robots.txt: It defines what part of the site can be crawled.
- Request Permission: If unsure, ask the website owner for permission to scrape.
2. Avoiding Overloading Servers
Sending too many requests too quickly will overload a server. This affects website performance and user experience.
- Rate Limiting: Use delays between requests to avoid overwhelming the server.
- Respect API Limits: If a website has an API, use it instead of scraping the site directly.
Example:
1 | import time |
By following these practices, you’ll be a responsible web scraper.
Final Thoughts
BeautifulSoup is excellent for web scraping. You can extract data from HTML and XML documents easily. Whether you’re scraping for analysis, research, or any other project, it is a simple but effective means of interacting with web content.
Respecting website rules and not overloading the server is a must while scraping. By learning how to use BeautifulSoup responsibly and adequately, you can create efficient and ethical web scraping scripts.
With practice, you can learn more advanced techniques to enhance your web scraping projects. Always check the website’s terms, handle data correctly, and be mindful of performance to get the most out of your web scraping experience.
Frequently Asked Questions
Q. What is web scraping with BeautifulSoup?
Web scraping with BeautifulSoup involves the use of the BeautifulSoup Python Library to collect information from the Web. It assists in parsing XML or HTML documents and allows the users to move through the contents to locate and retrieve the needed information, such as text, images, or links.
Q. Is BeautifulSoup legal?
Web scraping with BeautifulSoup is legal in most cases but depends on the website and how you use the data. Always review the website’s terms of service and the robots.txt file to make sure you’re not breaking any rules. Never scrape in a way that infringes on privacy or overloads the server.
Q. How do I handle dynamic content while scraping?
Dynamic content is content loaded by JavaScript, so it’s hard to scrape with BeautifulSoup. To scrape dynamic content, you may need to use additional tools like Selenium or Puppeteer, which simulate browser actions and load the JavaScript before scraping the content.