Web scraping is a powerful method for extracting data from websites, but transforming messy HTML into clean, structured information presents a significant challenge. That’s where Perplexity AI comes in. With AI, you can extract data faster and more accurately.
In this blog, we’ll show you how to use Perplexity AI for web scraping in Python. You’ll learn how to fetch HTML content, convert it to Markdown for better readability, and use AI to extract the data you need. We’ll also show you how Crawlbase Smart Proxy helps you avoid blocks and captchas while scraping protected websites. You can sign up now and get 5,000 free credits.
This blog is for developers, analysts, or anyone who wants to scrape the web smarter.
📚 Table of Contents
- Install Python
- Create a Virtual Environment
- Install Required Libraries
- Setup Perplexity API Access
- Send Requests and Parse HTML
- Convert HTML to Markdown for AI Processing
- How to Format Prompts
- Extract Key Details from Markdown
- Complete Code
- Challenges and Limitations of Perplexity AI in Web Scraping
- Avoid Getting Blocked: Use Crawlbase Smart Proxy
- Final Thoughts
- Frequently Asked Questions
Why Use Perplexity AI for Web Scraping?
Traditional web scraping uses Python libraries such as requests and BeautifulSoup to extract data from a website’s HTML. Works well for simple sites but becomes challenging when the HTML is messy or complex.
That’s where Perplexity AI comes in.
Perplexity AI is a smart tool that understands natural language and can find structured data inside raw HTML content. When you combine it with web scraping, it’s easier to extract clean, helpful, and organized data.
Perplexity AI for Scraping Benefits:
- Extract data from complex web pages
- Reduces time spent on writing custom parsing logic
- Works with Markdown-formatted HTML, which makes data extraction more accurate
- Returns structured output like JSON
By using Perplexity AI for web scraping in Python, you’ll scrape faster, smarter, and more efficiently.
Setting Up Your Python Environment
Before using Perplexity AI for web scraping, we need to prepare our Python environment. This setup ensures that everything runs smoothly and helps avoid errors later on.
✅ Install Python
If you haven’t already, install Python from the official website. Python is the primary language we’ll use to send requests, process web data, and talk to the Perplexity API.
✅ Create a Virtual Environment
A virtual environment keeps your project dependencies organized and avoids conflicts with other Python projects.
Open your terminal or command prompt and run:
1 | python -m venv perplexity_env |
Then activate the environment:
- Windows:
1 | perplexity_env\Scripts\activate |
- macOS/Linux:
1 | source perplexity_env/bin/activate |
✅ Install Required Libraries
Now, let’s install the Python packages we need:
1 | pip install requests beautifulsoup4 markdownify openai |
requests
: to send HTTP requestsbeautifulsoup4
: to parse HTMLmarkdownify
: to convert HTML to markdownopenai
: to connect with the Perplexity API (uses OpenAI-compatible format)
✅ Setup Perplexity API Access
To use Perplexity for web scraping, you need an API key. Perplexity offers an OpenAI-compatible API, which means you can use the same code format as OpenAI’s GPT models.
Here’s how to set it up:
- Get your API key from your Perplexity account dashboard.
- Set your API key in your code like this:
1 | from openai import OpenAI |
Ensure that you keep your API key secure and never share it publicly in code.
Step-by-Step Guide to Using Perplexity AI for Web Scraping
In this section, we’ll show you how to build a Python web scraper using Perplexity AI. You’ll learn how to scrape a web page, clean the content, convert it to Markdown, and use Perplexity AI to extract the data. We’ll use BeautifulSoup to select only the necessary part of the page, avoiding extra HTML that could increase costs by using more tokens.
We’ll use the following URL as an example:
1 | https://books.toscrape.com/catalogue/a-light-in-the-attic_1000/index.html |
🔹 Send Requests and Parse HTML
First, we need to send an HTTP request to the website and load the HTML content. Here’s how to do that using Python:
1 | import requests |
This code sends a request to a book’s webpage using requests
, gets the HTML content, and then uses BeautifulSoup
to parse that HTML so you can easily extract information from it
🔹 Convert HTML to Markdown for AI Processing
Perplexity AI performs better when we send clean, simplified text instead of raw HTML. To achieve this, we’ll use the markdownify
library to convert HTML into Markdown format. Sending only the relevant section reduces token usage and improves the quality of AI responses.
1 | from markdownify import markdownify as md |
The Markdown format is clean and easy for Perplexity AI to process, helping it focus on the important content.
🔹 How to Format Prompts
To achieve the best results with Perplexity AI, provide clear instructions (prompts). These prompts help the AI understand what you want to extract.
Here’s an example prompt:
1 | prompt = [ |
This prompt instructs the AI on exactly what to extract from the content.
🔹 Extract Key Details from Markdown
Now, let’s send this prompt to Perplexity AI using their OpenAI-compatible API:
1 | from openai import OpenAI |
🔹 Complete Code
Here’s the full working example combining everything:
1 | import requests |
Example Output:
1 | { |
Challenges and Limitations of Perplexity AI in Web Scraping
While Perplexity AI offers powerful features for web scraping, it does come with some challenges:
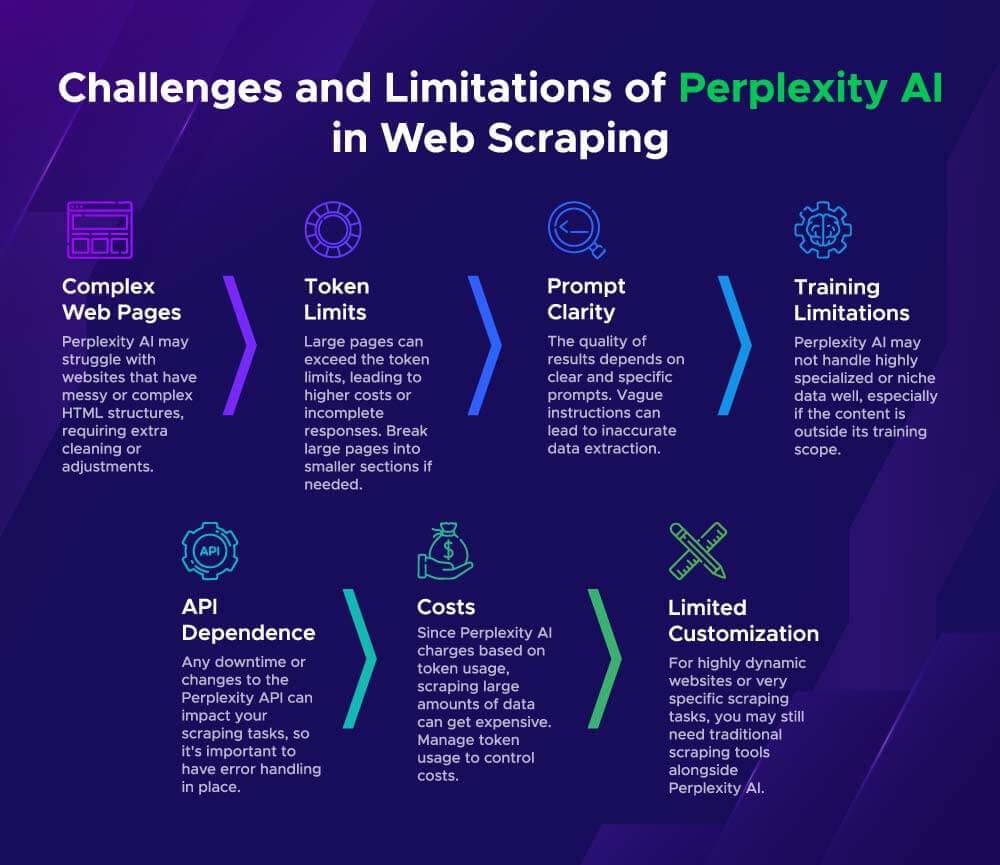
Understanding these limitations helps you maximize the benefits of Perplexity AI for web scraping while minimizing potential issues.
Avoid Getting Blocked: Use Crawlbase Smart Proxy
When scraping with Perplexity AI, websites often block bots, making it more challenging to obtain data. Crawlbase Smart Proxy solves this by rotating IP addresses and bypassing CAPTCHAs, allowing you to scrape websites without being blocked.
Why Use Crawlbase Smart Proxy with Perplexity AI?
- Bypass IP Blocks: Rotates IP addresses to avoid detection.
- Solve CAPTCHAs: Automatically handles CAPTCHAs, so you don’t have to.
- Save Time: No need to manage proxy servers—Crawlbase does it all.
- Clean HTML: Returns ready-to-use HTML for Perplexity AI.
Example Code:
1 | import requests |
With Crawlbase Smart Proxy, you can scrape websites safely, bypass blocks, and get clean data for processing with Perplexity AI.
Final Thoughts
Utilizing Perplexity AI for web scraping in Python can enhance your scraping tasks by making them faster, smarter, and more accurate. By converting raw HTML to Markdown and utilizing AI to extract structured data, you can streamline your process and save time.
However, scraping websites can be challenging, especially when encountering blocks and CAPTCHAs. That’s where Crawlbase Smart Proxy comes in. It helps you avoid IP blocks and solves CAPTCHAs, allowing you to scrape websites without interruptions. This combination of Perplexity AI and Crawlbase Smart Proxy makes web scraping more efficient and scalable, allowing you to obtain the data you need without being blocked.
Frequently Asked Questions
Q. What is Perplexity AI, and how does it help with web scraping?
Perplexity AI is a tool that uses natural language processing to help you extract structured data from raw HTML content. It makes scraping easier by converting messy HTML into readable Markdown and extracting key details with AI. Saves you time and improves data extraction accuracy.
Q. How does Crawlbase Smart Proxy prevent my scraper from getting blocked?
Crawlbase Smart Proxy rotates IP addresses and solves CAPTCHAs, making it appear as if a real user is browsing the site. It helps avoid IP blocks and lets you scrape websites without being detected as a bot. A reliable tool to keep your scraping tasks running.
Q. Can I use Perplexity AI and Crawlbase Smart Proxy together?
Yes! Using Perplexity AI for data extraction and Crawlbase Smart Proxy for bypassing blocks and CAPTCHAs is a killer combo. Crawlbase enables seamless access to the website, and Perplexity AI facilitates the cleaning and processing of data.