If you’re involved in crypto trading, building dashboards, or conducting market research, obtaining real-time cryptocurrency prices is crucial. While CoinMarketCap provides a significant amount of this data on its website, it does not offer a free and open API for live prices. That’s where web scraping becomes useful.
In this article, we’ll show you how to scrape live crypto prices from CoinMarketCap using Python. We’ll guide you through sending requests, parsing HTML, and extracting data for popular cryptocurrencies like Bitcoin, Ethereum, and other top coins. You’ll also learn how to avoid getting blocked by using tools like Crawlbase Smart Proxy and how to export your data for future use.
Let’s get started.
Table of Contents
- Python & Required Libraries
- Installing BeautifulSoup and Requests
- Send HTTP Request to CoinMarketCap
- Parse the HTML with BeautifulSoup
- Extract Live Crypto Prices
- Avoid Getting Blocked: Use Crawlbase Smart Proxy
- Export the Data to CSV or JSON
- Final Thoughts
- Frequently Asked Questions
Why Scrape CoinMarketCap for Crypto Prices?
CoinMarketCap is the most popular website for tracking cryptocurrency prices, market cap, volume, and rankings. It updates prices in real-time and has thousands of coins. It’s the go-to source for crypto data.
However, CoinMarketCap’s official API has limits on free access, and real-time data requires a paid plan. If you’re a developer, researcher, or crypto enthusiast looking for free and flexible access to live price data, web scraping is the way to go.
Scraping cryptocurrency prices from CoinMarketCap can be very useful for various purposes. The following image illustrates some common use cases with their corresponding examples for Crypto Price Scraping.
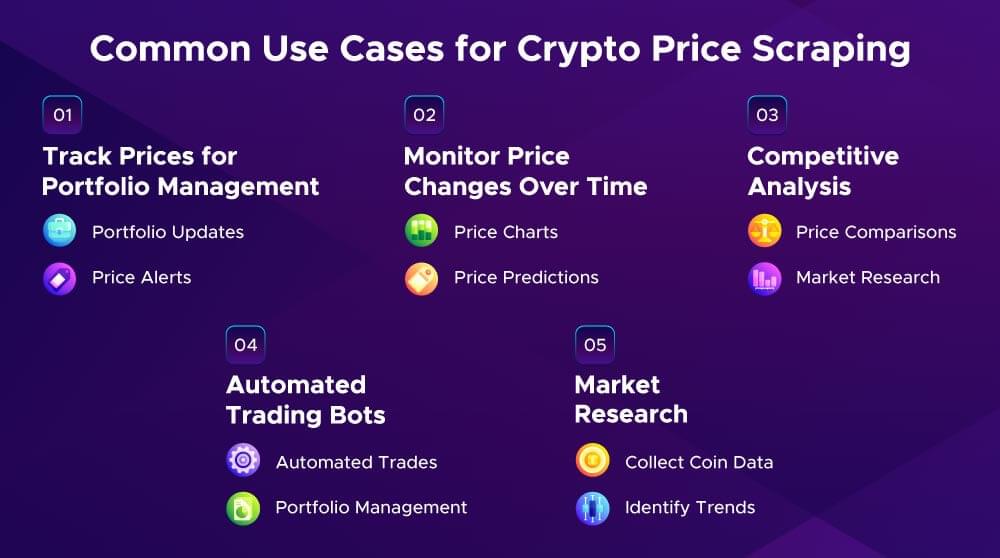
With Python, BeautifulSoup, and smart proxies, you can extract this data easily and reliably without paying for expensive APIs.
Tools You Need to Get Started
Before we scrape crypto prices from CoinMarketCap, let’s set up the tools and libraries we need. We’ll use Python because it’s simple, powerful, and has great libraries for web scraping.
Python & Required Libraries
Make sure you have Python installed. You can download it from python.org.
We’ll use two main Python libraries:
- Requests – to send HTTP requests and get the HTML of the page
- BeautifulSoup – to parse and extract data from the HTML
🔧 Installing BeautifulSoup and Requests
You can install both libraries using pip, which is Python’s package manager. Open your terminal or command prompt and run:
1 | pip install requests beautifulsoup4 |
Once installed, you’re ready to start scraping crypto data from CoinMarketCap.
Step-by-Step Guide to Scrape Crypto Prices on CoinMarketCap
Now that your setup is ready, let’s scrape real-time cryptocurrency prices from CoinMarketCap. We’ll go step by step using Python and BeautifulSoup.
Send HTTP Request to CoinMarketCap
First, we need to send a request to the CoinMarketCap website and get the page content.
1 | import requests |
Always use headers like User-Agent to mimic a real browser.
Parse the HTML with BeautifulSoup
Once we have the page content, we can parse it using BeautifulSoup.
1 | from bs4 import BeautifulSoup |
Extract Live Crypto Prices from CoinMarketCap
Let’s extract the name and current price of the top 10 cryptocurrencies.
CoinMarketCap uses dynamic HTML classes, so you may need to inspect the page source to adjust the class names.
Here’s a working example:
1 | # Find the table rows that contain the coins |
Output:
1 | Bitcoin: $92,477.64 |
Note: Class names are subject to frequent changes. You can always right-click → “Inspect” on the page to check the updated class names
Avoid Getting Blocked: Use Crawlbase Smart Proxy
CoinMarketCap uses protection like Cloudflare to block bots. If you send too many requests, your IP can get blocked. To fix this, use Crawlbase Smart Proxy. It helps you scrape data without getting blocked or dealing with captchas. Additionally, you don’t need to worry about manually rotating IPs, as it’s all handled for you.
How to Use Crawlbase Smart Proxy in Python
Here’s a simple example using requests
:
1 | import requests |
You can get a token by signing up on Crawlbase. With Crawlbase Smart Proxy, your scraper stays stable and unblocked—even on sites with strong protections.
Exporting the Data to CSV or JSON
In this section, we’ll see how to export cryptocurrency data from CoinMarketCap to both CSV and JSON formats. This is useful when you want to store the data or analyze it later. We will also use Crawlbase Smart Proxy to prevent being blocked while scraping.
Steps:
- Use Crawlbase Smart Proxy to send requests to CoinMarketCap.
- Extract cryptocurrency data, including name, symbol, and price.
- Export the data to CSV and JSON formats.
Here’s the complete code to accomplish this:
1 | import requests |
This way, you can scrape data from CoinMarketCap without getting blocked, and the data export feature makes it easy to save and analyze later. If you need to scrape more data or automate the process, just adjust the logic accordingly.
Final Thoughts
Scraping cryptocurrency prices from CoinMarketCap using Python is a smart way to stay up-to-date with the volatile financial markets. With just a few lines of code, you can track live prices, export data, and use it for analysis or building crypto tools.
However, remember that websites like CoinMarketCap have built-in anti-bot protections. That’s why using tools like Crawlbase Smart Proxy is very helpful. It keeps your scraping smooth and avoids IP blocks and captchas.
Whether you’re a developer, trader, or data analyst, this guide gives you a simple way to get started with crypto data scraping using Python. Sign up now.
Frequently Asked Questions
Q. Is it legal to scrape CoinMarketCap for crypto prices?
Scraping public data from CoinMarketCap is generally allowed for personal or educational use. However, always check their Terms of Service before using scraped data for commercial projects to avoid any legal issues.
Q. Why do I get blocked while scraping CoinMarketCap?
CoinMarketCap utilizes security tools, such as Cloudflare, to block bots. If you send too many requests from the same IP address, you may face CAPTCHAs or blocks. To solve this, use Crawlbase Smart Proxy,, which automatically rotates IPs and bypasses bot protection.
Q. How often can I scrape crypto prices?
If you’re scraping manually, it’s safe to fetch data every few minutes to avoid getting blocked. With Crawlbase Smart Proxy, you can scale scraping with better speed and frequency, making it ideal for tracking live crypto data.