It can be tedious to find the best prices online when so many stores sell the same product for different prices. Instead of checking each site manually, web scraping lets you scrape prices from multiple sites in seconds which you can compare to find the best deal.
In this tutorial, we’ll go through creating a Python script that reads data including prices from a JSON file and finds the lowest price. From setting up your environment and understanding the data formats to writing a script that can find the best deals, we’ll go through each step. By the end, you’ll have a working solution that will save you time and money.
Let’s explore how to use web scraping for effective price comparison.
Table of Contents
- Installing Python and Required Libraries
- JSON Data Source
- Loading JSON Data
- Getting Price Data from Different Stores
- Finding the Cheapest
- Complete Code Example
- Handling Dynamic Content
- Choosing a Proxy
Why Use Web Scraping for Price Comparison?
With online shopping becoming more popular, finding the best price for a product across multiple stores has become essential. Manually checking each store can be a pain. Web scraping is a way to automate this process, so you can scrape prices from multiple websites in seconds.
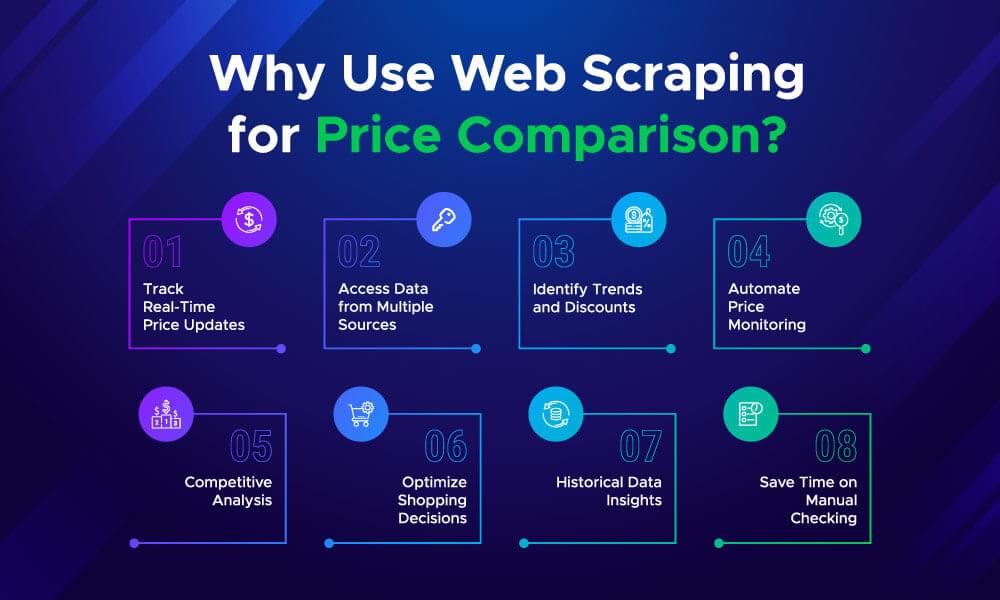
Using web scraping for price comparison not only saves time but also helps you make smarter buying decisions. By extracting prices from various sources, you get a clear view of where the best deals are. Web scraping can be set up to update prices regularly, so you’re always working with the latest information.
This is especially helpful for businesses tracking competitors, individuals looking for deals or anyone who wants to compare product prices quickly. In this guide, we’ll show you how to set up a simple Python based web scraper to compare prices from various sources.
Whether you’re a beginner or have some coding experience, web scraping for price comparison is a skill that will save you time and money.
Setting Up the Environment
Before starting with the price comparison script, we need to set up the environment. In this section we will cover the basics of getting Python and required libraries installed and a JSON file to use as a data source. This will make it easy to manage and run your price comparison script.
Installing Python and Required Libraries
Make sure you have Python installed on your computer. Python is used for web scraping because it’s easy and has great libraries. You can download the latest version from the official Python website.
Once Python is installed we will need a few libraries to make web scraping and data processing easier. Open your terminal and install these libraries using the following commands:
1 | pip install requests |
**requests**
: This library helps us make HTTP requests to websites, so we can fetch webpage data.**json**
: This module is built into Python and helps us work with JSON files, which is a common data format to store and exchange data.
These libraries will set up a basic environment for web scraping and data manipulation.
JSON Data Source
For this tutorial, we’ll use a JSON file as a data source. This JSON will mimic data from different online stores, including prices for the same product at different stores. A sample JSON looks like this:
1 | { |
This JSON allows us to compare prices for multiple products across multiple stores. Each product has a name and each store has a price for the product.
In the following sections, we’ll go over how to load this JSON data, get prices and find the best deals. This data source will make our script neat and easy to update as we go.
Writing the Price Comparison Script in Python
Now let’s write a Python script to compare product prices across multiple stores. This script will load and parse the JSON data, get prices for each product, and find the store with the lowest price.
Loading JSON Data
First, we’ll load the JSON data into our script. Python’s json
module makes it easy to read and work with JSON. Here’s a basic example of loading the file.
1 | import json |
This code reads the JSON file products.json
and loads it into a Python dictionary called data. You can use print(data)
to see if the JSON data is structured correctly.
Getting Price Data from Different Stores
Now, we’ll loop through each product to get its stores
list, where prices from different stores are available. For each product we’ll get the store name and price for easier comparison.
1 | for product in data['products']: |
This will show each product’s name, store name and price so you can see everything is correctly loaded.
Finding the Cheapest
To find the lowest price, we’ll create a small function that iterates through the stores for each product and picks the store with the minimum price. We can store this information for each product to summarize later.
To find the cheapest, we’ll create a small function that loops through the stores for each product and picks the store with the cheapest price.
1 | def find_lowest_price(product): |
Now, let’s use this function for each product in our data.
1 | for product in data['products']: |
Complete Code Example
Putting it all together, here’s the full code to load the JSON, extract prices and display the store with the best price for each product.
1 | import json |
This script will print the product name and the store with the lowest price for each item so you can see where to buy each product at the best price.
Example Output:
1 | Product: Smartphone XYZ |
Best Practices for Web Scraping Price Data
When scraping prices follow best practices for better performance and reliability.
Handling Dynamic Content
Many sites load content via JavaScript which standard scraping will miss. To handle this:
- Selenium or Puppeteer: These tools simulate a real browser to load dynamic content.
- Leverage APIs: Some sites have AJAX endpoints you can access directly for data which is often faster.
- Use Third-Party Solutions: Services like Crawlbase Crawling API handle JavaScript heavy sites and can simplify scraping, reduce the risk of being blocked.
Choosing a Proxy
Proxies help with blocks, especially for frequent requests.
- Residential Proxies mimic real users, low detection risk but expensive.
- Rotating Proxies switch IPs with each request, good for high volume.
- Crawlbase Smart Proxy handles proxy rotation and IP management, so your scraper stays undetected and has a fast connection. Good for high volume scraping and avoiding IP ban.
Follow these and you won’t get blocked and your scraper will run.
Final Thoughts
Web scraping for price comparison is a powerful tool to gather up to date product prices from different stores. It saves you time and helps you make better buying decisions by doing the process auto-magically.
In this blog, we covered how to set up your environment, work with JSON data, and write a price comparison script. We also mentioned best practices including using third-party solutions like Crawlbase Crawling API or Crawlbase Smart Proxy for smoother and more reliable scraping.
By following these steps, you’ll be able to scrape price data in no time. Keep testing and perfecting your scrape for the best results. Happy scraping!
Frequently Asked Questions
Q. What is web scraping, and why should I use it for price comparison?
Web scraping is a way to automatically extract data from websites. For price comparison, it allows you to collect real-time pricing information from multiple online stores so you can find the best deals. By automating this process, you’ll save time and make better buying decisions.
Q. How do I handle dynamic content while web scraping?
Dynamic content, like data loaded through JavaScript, can be tough to scrape. To handle it, you can use tools like Crawlbase Crawling API, which can bypass JavaScript restrictions and help you collect data more reliably. This way you get accurate and up-to-date information even from websites with complex loading mechanisms.
Q. What are the best practices for web scraping?
Some of the best practices for web scraping are respecting website terms of service, using proxies to avoid getting blocked and handling errors in your script. Using third-party services like Crawlbase for dynamic content and rotating proxies will make your scrape smooth and efficient and won’t get you blocked.